怎么用java代码输出一个会动的爱心
时间: 2023-09-18 17:05:38 浏览: 102
### 回答1:
您好,以下是使用Java代码输出一个动态的爱心的示例:
```java
public class AnimatedHeart {
public static void main(String[] args) throws InterruptedException {
String heart = " ** **\n **** ****\n**********\n ********\n ******\n ****\n **";
int width = 11;
int height = 7;
int x = 0;
int y = 0;
// Infinite loop for animation
while (true) {
// Clear the console screen
new ProcessBuilder("cmd", "/c", "cls").inheritIO().start().waitFor();
// Move the heart to the next position
x++;
y = (int) (15 * Math.sin(x * 0.1) - height / 2);
// Draw the heart at the current position
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (i == y && j == x % (width - 1))
System.out.print(heart.charAt(i * (width + 1) + j));
else
System.out.print(" ");
}
System.out.println();
}
// Pause the animation for 100 milliseconds
Thread.sleep(100);
}
}
}
```
运行这段代码,您将看到一个动态的爱心在控制台屏幕上跳动。
### 回答2:
要用Java代码输出一个会动的爱心,可以通过绘制图形来实现。下面是一个简单的示例:
```java
import java.awt.*;
import javax.swing.*;
public class AnimatedHeart extends JPanel {
private int x;
private int y;
private int dx;
private int dy;
public AnimatedHeart() {
x = 200;
y = 200;
dx = 2;
dy = 2;
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// 绘制红色心形
g2d.setColor(Color.RED);
g2d.fillArc(x, y, 100, 100, 0, 180);
g2d.fillArc(x + 50, y, 100, 100, 0, 180);
g2d.fillRect(x, y + 50, 150, 100);
// 更新心形位置
x += dx;
y += dy;
// 碰到边界时反向移动
if (x < 0 || x > this.getWidth() - 150) {
dx = -dx;
}
if (y < 0 || y > this.getHeight() - 100) {
dy = -dy;
}
// 重绘心形
repaint();
}
public static void main(String[] args) {
JFrame frame = new JFrame("Animated Heart");
AnimatedHeart heart = new AnimatedHeart();
frame.add(heart);
frame.setSize(500, 500);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
```
以上代码使用Java的图形库绘制了一个红色的心形,并通过改变心形的位置来实现动画效果。程序运行后会弹出一个窗口,其中显示了一个会动的爱心。爱心会在窗口内不断移动,碰到窗口边界时会反向移动。由于代码中使用了repaint()方法,每次心形位置更新后都会立即重绘,从而实现动态效果。
### 回答3:
要用Java代码输出一个会动的爱心,可以使用图形库来绘制爱心的形状,然后在每次循环中改变爱心的位置,使其看起来会动。
首先,我们需要导入Java图形库(如java.awt库)来绘制图形。接下来,创建一个继承自JFrame的类来显示我们的动态爱心。
在这个类中,我们需要重写paint方法来绘制动态爱心的形状。可以使用Graphics类的方法来绘制一个心形图形,例如drawArc和fillArc方法来绘制心形的两个半圆,drawLine方法来绘制心形的尖端和底部。
然后,在主函数中创建我们定义的类的实例,设置窗口大小,并使窗口可见。
接下来,我们需要在一个循环中不断改变爱心的位置,使其看起来会动。可以使用线程来控制频率。在每次循环中,可以调用repaint方法来重绘爱心,同时改变爱心的位置(通过更新爱心的坐标)。
具体的伪代码如下所示:
```java
import java.awt.*;
import javax.swing.*;
class AnimatedHeart extends JFrame {
private int x, y; // 爱心的坐标
public AnimatedHeart() {
setSize(400, 400); // 设定窗口大小
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void paint(Graphics g) {
// 绘制动态爱心的形状
g.setColor(Color.RED);
g.fillArc(x, y, 100, 100, 45, 180);
g.fillArc(x + 50, y, 100, 100, 45, 180);
g.drawLine(x + 25, y + 80, x + 150, y + 80);
g.drawLine(x + 25, y + 80, x - 100, y + 80);
}
public static void main(String[] args) {
AnimatedHeart heart = new AnimatedHeart();
while (true) {
// 更新爱心的坐标,使其看起来会动
heart.x += 5;
heart.y += 5;
if (heart.x > 400) {
heart.x = 0;
heart.y = 0;
}
try {
Thread.sleep(100); // 控制动画的速度
} catch (InterruptedException e) {
e.printStackTrace();
}
heart.repaint(); // 重绘爱心
}
}
}
```
以上代码的核心思想是在每个循环中更新爱心的坐标,并通过repaint方法来重绘爱心,从而实现爱心的动态效果。可以根据实际需求调整爱心的大小、颜色、速度等参数来得到所需的动态爱心效果。
阅读全文
相关推荐
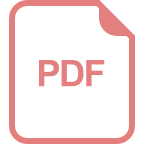





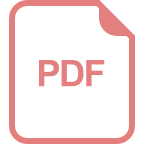
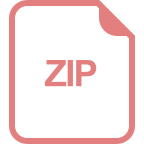
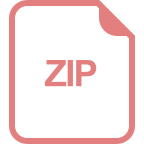
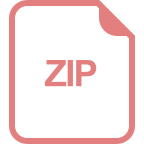
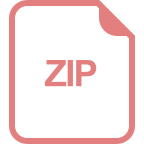
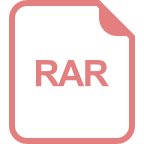
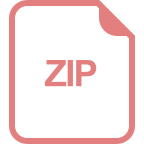
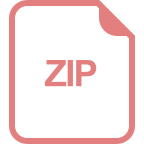