具有三个输入因素的BP神经网络模型预测的python代码
时间: 2024-01-26 12:01:43 浏览: 79
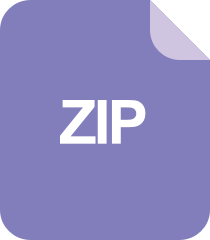
基于BP神经网络的数据回归预测Python代码 使用excel数据集 numpy实现

以下是一个三个输入因素的BP神经网络模型的Python代码示例:
```python
import numpy as np
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义BP神经网络类
class BPNN:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 初始化权重矩阵
self.W1 = np.random.randn(input_size, hidden_size)
self.W2 = np.random.randn(hidden_size, output_size)
# 初始化偏差值
self.b1 = np.zeros((1, hidden_size))
self.b2 = np.zeros((1, output_size))
# 前向传播函数
def forward(self, X):
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
self.y_hat = sigmoid(self.z2)
return self.y_hat
# 反向传播函数
def backward(self, X, y_true, y_hat, learning_rate):
delta2 = (y_hat - y_true) * y_hat * (1 - y_hat)
dW2 = np.dot(self.a1.T, delta2)
db2 = np.sum(delta2, axis=0, keepdims=True)
delta1 = np.dot(delta2, self.W2.T) * self.a1 * (1 - self.a1)
dW1 = np.dot(X.T, delta1)
db1 = np.sum(delta1, axis=0)
# 更新权重和偏差值
self.W2 -= learning_rate * dW2
self.b2 -= learning_rate * db2
self.W1 -= learning_rate * dW1
self.b1 -= learning_rate * db1
# 训练函数
def train(self, X, y_true, learning_rate, epochs):
for i in range(epochs):
y_hat = self.forward(X)
self.backward(X, y_true, y_hat, learning_rate)
if i % 100 == 0:
loss = np.mean(np.square(y_hat - y_true))
print("Epoch:", i, "Loss:", loss)
```
使用方法:
```python
# 构建数据集
X = np.array([[0.1, 0.2, 0.3], [0.2, 0.3, 0.4], [0.3, 0.4, 0.5], [0.4, 0.5, 0.6]])
y = np.array([[0.4], [0.5], [0.6], [0.7]])
# 创建BP神经网络模型对象
bpnn = BPNN(input_size=3, hidden_size=4, output_size=1)
# 训练模型
bpnn.train(X, y, learning_rate=0.1, epochs=1000)
# 使用模型进行预测
X_test = np.array([[0.5, 0.6, 0.7]])
y_pred = bpnn.forward(X_test)
print("预测值:", y_pred)
```
阅读全文
相关推荐
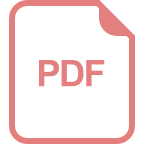
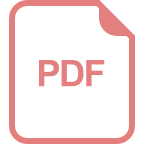
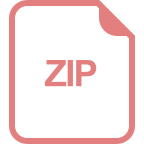
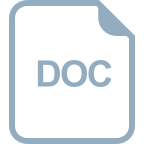
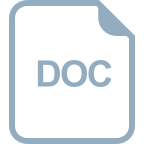
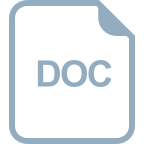
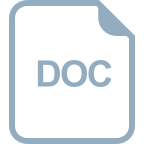








