python将一个文本去除符号
时间: 2024-03-08 15:47:24 浏览: 68
可以使用Python的字符串操作和正则表达式来去除文本中的符号。下面给出一个简单的示例代码:
```python
import re
text = "Hello, world! This is a sample text."
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
print(text)
```
输出结果为:
```
Hello world This is a sample text
```
在这个示例中,`re.sub`函数使用正则表达式`r'[^\w\s]'`匹配所有非字母数字和空格字符,然后将它们替换为空字符串。这样就可以去除文本中的标点符号了。
相关问题
python去除文本的标点符号
在Python中,你可以使用`string`模块的`punctuation`常量,结合循环或者列表推导式来去除文本中的标点符号。以下是一个简单的例子:
```python
import string
def remove_punctuation(text):
# 获取所有的标点符号
punctuation_chars = string.punctuation
# 使用列表推导式或循环创建一个新的字符串,排除标点符号
no_punct_text = ''.join(char for char in text if char not in punctuation_chars)
return no_punct_text
# 测试
text_with_punctuation = "Hello, world! This is a test sentence."
no_punctuation_text = remove_punctuation(text_with_punctuation)
print("去除标点后的文本:", no_punctuation_text)
```
在这个例子中,我们从输入文本中过滤出不属于`string.punctuation`中的字符,从而得到一个不含标点符号的新字符串。
python处理txt文本的标点符号
Python处理txt文本的标点符号可以使用Python的re模块中的正则表达式来实现。以下是一个示例代码:
```python
import re
# 打开文本文件
with open('example.txt', 'r') as f:
text = f.read()
# 使用正则表达式去掉标点符号
text = re.sub(r'[^\w\s]', '', text)
# 输出处理后的文本
print(text)
```
上述代码使用了re.sub()函数,它接受三个参数:正则表达式、替换字符串和要处理的文本。正则表达式`[^\w\s]`表示匹配除了字母、数字和空格之外的任何字符,然后将其替换为空字符串,从而去掉了标点符号。
阅读全文
相关推荐
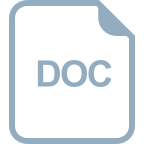
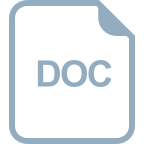
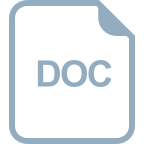













