写一个java程序,并写好注释 背景:某城市新建一座野生动物园,其中包括三个馆:哺乳动物馆,两栖爬行动物馆与海洋馆。每个馆有饲养员一名,动物园设馆长一名。 场景:有一批动物(狮子,老虎,鳄鱼,蝾螈,海豚,乌贼)需要进驻动物园,由馆长负责调度进馆,有管理员负责饲养与展出。 要求:用java面向对象思想编写该场景中各个对象的类与接口。
时间: 2023-06-04 21:05:01 浏览: 185
把类与接口都写好注释:
//动物接口
public interface Animal {
public String getType(); //获取动物类型
public void display(); //展示动物
public void feed(); //喂养动物
}
//哺乳动物类
public class Mammal implements Animal {
private String type;
private String name;
private String color;
private String gender;
private String food;
public Mammal(String name, String color, String gender, String food) {
this.type = "哺乳动物";
this.name = name;
this.color = color;
this.gender = gender;
this.food = food;
}
public String getType() {
return this.type;
}
public void display() {
System.out.println("我是一只" + this.color + "色的" + this.type + ",我的名字叫" + this.name + ",性别是" + this.gender);
}
public void feed() {
System.out.println("饲养员正在喂食" + this.name + ",给它吃" + this.food + "。");
}
}
//两栖爬行动物类
public class AmphibianAndReptile implements Animal {
private String type;
private String name;
private String color;
private String gender;
private String food;
public AmphibianAndReptile(String name, String color, String gender, String food) {
this.type = "两栖爬行动物";
this.name = name;
this.color = color;
this.gender = gender;
this.food = food;
}
public String getType() {
return this.type;
}
public void display() {
System.out.println("我是一只" + this.color + "色的" + this.type + ",我的名字叫" + this.name + ",性别是" + this.gender);
}
public void feed() {
System.out.println("饲养员正在喂食" + this.name + ",给它吃" + this.food + "。");
}
}
//海洋动物类
public class MarineAnimals implements Animal {
private String type;
private String name;
private String color;
private String gender;
private String food;
public MarineAnimals(String name, String color, String gender, String food) {
this.type = "海洋动物";
this.name = name;
this.color = color;
this.gender = gender;
this.food = food;
}
public String getType() {
return this.type;
}
public void display() {
System.out.println("我是一只" + this.color + "色的" + this.type + ",我的名字叫" + this.name + ",性别是" + this.gender);
}
public void feed() {
System.out.println("饲养员正在喂食" + this.name + ",给它吃" + this.food + "。");
}
}
//饲养员类
public class CareTaker {
private String name;
private Animal animal;
public CareTaker(String name, Animal animal) {
this.name = name;
this.animal = animal;
}
public void feedAnimal() {
animal.feed();
}
}
//馆长类
public class Zookeeper {
private String name;
private List<Animal> animals;
public Zookeeper(String name) {
this.name = name;
animals = new ArrayList<Animal>();
}
public void addAnimal(Animal animal) {
animals.add(animal);
System.out.println("馆长调度好了一只" + animal.getType() + ",让它进入了动物园。");
}
public void showAnimals() {
for (Animal animal : animals) {
animal.display();
}
}
}
//主函数类
public class Main {
public static void main(String[] args) {
//新建饲养员
CareTaker mammalCareTaker = new CareTaker("张三", new Mammal("狮子", "黄", "雄性", "肉"));
CareTaker AmphibianAndReptileCareTaker = new CareTaker("李四", new AmphibianAndReptile("蝾螈", "黑", "雌性", "虫子"));
CareTaker marineAnimalsCareTaker = new CareTaker("王五", new MarineAnimals("乌贼", "灰", "雄性", "鱼"));
//新建馆长
Zookeeper zookeeper = new Zookeeper("赵六");
//馆长调度动物进馆
zookeeper.addAnimal(new Mammal("老虎", "橙", "雌性", "肉"));
zookeeper.addAnimal(new AmphibianAndReptile("蝎子", "棕", "雄性", "虫子"));
zookeeper.addAnimal(new MarineAnimals("海豚", "白", "雌性", "鱼"));
//馆长展示动物
zookeeper.showAnimals();
//饲养员喂养动物
mammalCareTaker.feedAnimal();
AmphibianAndReptileCareTaker.feedAnimal();
marineAnimalsCareTaker.feedAnimal();
}
}
相关推荐
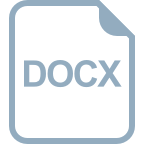
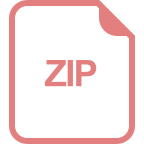














