c语言创建文本并写入内容查找相应内容并修改文本内容
时间: 2023-12-02 19:04:11 浏览: 108
要创建文本并写入内容,可以使用C语言的文件操作函数来实现。下面是一个简单的示例代码,可以创建一个名为"test.txt"的文件,并向其中写入一些内容:
```c
#include <stdio.h>
int main()
{
char filename[] = "test.txt";
char content[] = "Hello, world!";
FILE *fp;
fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to create file: %s\n", filename);
return -1;
}
fputs(content, fp);
fclose(fp);
return 0;
}
```
这个程序将创建名为"test.txt"的文件,并向其中写入"Hello, world!"字符串。如果文件创建失败,程序将返回-1。
要查找相应内容并修改文本内容,可以使用类似前面提到的示例代码,先打开文件并读取其中的内容,然后查找需要替换的字符串并进行替换,最后将修改后的内容写入文件中。下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BUF_SIZE 1024
int main()
{
char old_str[] = "hello";
char new_str[] = "world";
char filename[] = "test.txt";
char buf[MAX_BUF_SIZE];
char content[MAX_BUF_SIZE];
FILE *fp;
int found = 0;
fp = fopen(filename, "r+");
if (fp == NULL) {
printf("Failed to open file: %s\n", filename);
return -1;
}
while (fgets(buf, MAX_BUF_SIZE, fp) != NULL) {
char *p = strstr(buf, old_str);
if (p != NULL) {
found = 1;
sprintf(content, "%s%s%s", content, new_str, p + strlen(old_str));
fseek(fp, -(strlen(buf) - (p - buf + strlen(old_str))), SEEK_CUR);
fputs(content, fp);
fflush(fp);
break;
} else {
sprintf(content, "%s%s", content, buf);
}
}
if (!found) {
printf("Failed to find string: %s\n", old_str);
}
fclose(fp);
return 0;
}
```
这个程序将打开名为"test.txt"的文件,查找其中第一个出现的"hello"字符串,并将其替换成"world"字符串。如果文件不存在或读写失败,程序将返回-1。如果找不到需要替换的字符串,程序将输出一条错误消息。
阅读全文
相关推荐
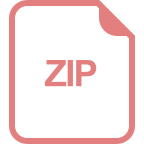
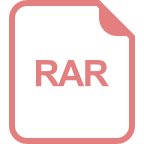
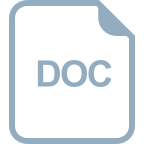
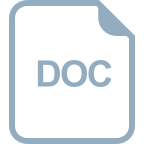
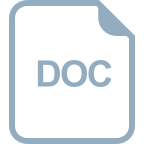
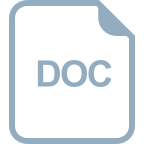
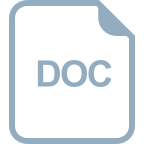
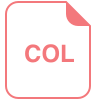
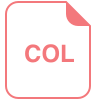

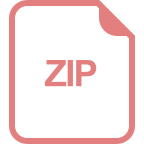
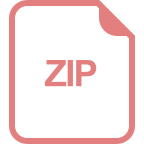
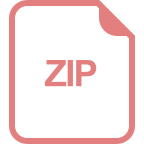
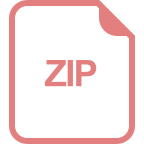

