how to split a docx file to docx files by title using docx4j
时间: 2024-04-08 10:29:03 浏览: 97
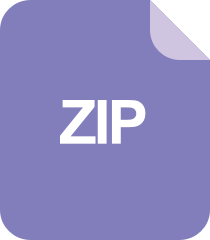
最新 docx4j-master
To split a DOCX file into multiple DOCX files by title using docx4j, you can follow these steps:
1. Load the original DOCX file using docx4j.
2. Iterate through the document's paragraphs and identify the paragraphs that represent titles/headings. You can use the paragraph's style or any other identifying feature to determine the titles.
3. For each title paragraph, create a new DOCX file.
4. Copy all the paragraphs from the original document until you encounter the next title paragraph. Add these paragraphs to the newly created DOCX file.
5. Save the newly created DOCX file.
Here's a sample code snippet to demonstrate this process:
```java
import org.docx4j.Docx4J;
import org.docx4j.openpackaging.packages.WordprocessingMLPackage;
import org.docx4j.wml.Body;
import org.docx4j.wml.Document;
import org.docx4j.wml.P;
import org.docx4j.wml.PPr;
import org.docx4j.wml.PStyle;
import java.io.File;
import java.util.List;
public class DocxSplitter {
public static void main(String[] args) {
try {
// Load the original DOCX file
WordprocessingMLPackage wordMLPackage = Docx4J.load(new File("input.docx"));
Document document = wordMLPackage.getMainDocumentPart().getJaxbElement();
Body body = document.getBody();
List<Object> contentList = body.getContent();
// Variables to track split
String currentTitle = null;
WordprocessingMLPackage currentPackage = null;
for (Object content : contentList) {
if (content instanceof P) {
P paragraph = (P) content;
PPr ppr = paragraph.getPPr();
if (ppr != null) {
PStyle pStyle = ppr.getPStyle();
if (pStyle != null) {
String style = pStyle.getVal();
if (style.equals("Title")) {
// Start a new DOCX file for the title
if (currentPackage != null) {
String fileName = currentTitle + ".docx";
currentPackage.save(new File(fileName));
}
currentTitle = paragraph.toString();
currentPackage = WordprocessingMLPackage.createPackage();
}
}
}
if (currentPackage != null) {
currentPackage.getMainDocumentPart().getContent().add(paragraph);
}
}
}
// Save the last package
if (currentPackage != null) {
String fileName = currentTitle + ".docx";
currentPackage.save(new File(fileName));
}
System.out.println("Splitting complete!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
Make sure to replace "input.docx" with the path to your actual input file. The code assumes that the title paragraphs have a style named "Title" applied. Adjust the code accordingly if your title paragraphs have a different style name.
阅读全文
相关推荐
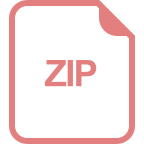
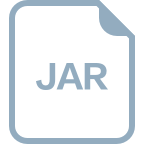
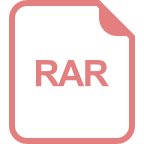
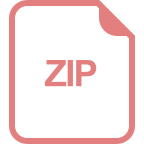
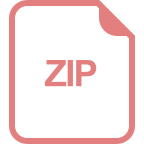
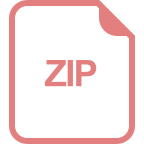
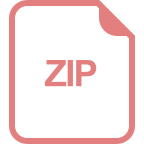
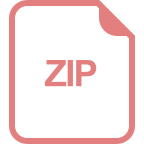
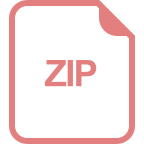
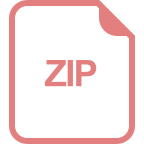
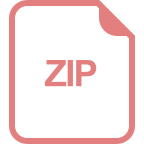
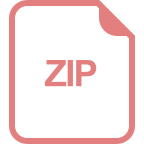