由随机梯度上升算法,改进成小批量梯度上升算法,python
时间: 2023-11-10 08:09:22 浏览: 34
小批量梯度上升算法是随机梯度上升算法的一种改进方法,其主要思想是将样本数据集分成若干个小批量,每次随机选择一个小批量的数据样本进行梯度上升更新参数,从而减少了随机梯度上升算法的参数更新抖动,提高了算法的稳定性和收敛速度。
下面是使用Python实现小批量梯度上升算法的示例代码:
```python
import numpy as np
# 定义sigmoid函数
def sigmoid(x):
return 1.0 / (1 + np.exp(-x))
# 定义小批量梯度上升算法函数
def batch_gradient_ascent(data, labels, batch_size, alpha, max_iter):
m, n = np.shape(data)
weights = np.ones((n, 1)) # 初始化回归系数为1
for i in range(max_iter):
# 随机选择一个小批量的数据样本
batch_index = np.random.choice(m, batch_size)
batch_data = data[batch_index]
batch_labels = labels[batch_index]
# 计算梯度
h = sigmoid(np.dot(batch_data, weights))
error = batch_labels - h
weights = weights + alpha * np.dot(batch_data.transpose(), error)
return weights
# 测试
data = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
labels = np.array([0, 0, 1, 1]).reshape(-1, 1)
batch_size = 2
alpha = 0.01
max_iter = 1000
weights = batch_gradient_ascent(data, labels, batch_size, alpha, max_iter)
print(weights)
```
在上面的代码中,我们首先定义了sigmoid函数,然后定义了小批量梯度上升算法函数batch_gradient_ascent,其中data和labels分别代表数据集和标签,batch_size代表每次更新参数时随机选择的小批量数据样本的大小,alpha代表学习率,max_iter代表最大迭代次数。在函数中,我们首先初始化回归系数weights为1,然后进行迭代更新,每次随机选择一个小批量的数据样本,计算梯度,更新回归系数。最后返回回归系数weights。
在测试代码中,我们定义了一个包含4个数据样本的数据集,其中前2个样本的标签为0,后2个样本的标签为1。我们将batch_size设置为2,alpha设置为0.01,max_iter设置为1000,最后调用batch_gradient_ascent函数进行模型训练,得到回归系数weights。
相关推荐
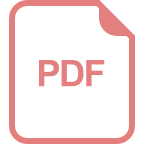
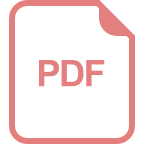














