esp32 idf ap tcp server
时间: 2023-08-27 17:05:13 浏览: 47
The ESP32 IDF (Integrated Development Framework) allows developers to create a TCP server on an ESP32 device. Here are the basic steps to create a TCP server:
1. Include the required libraries and initialize the network stack.
```c
#include <stdio.h>
#include <string.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_wifi.h"
#include "esp_event.h"
#include "esp_log.h"
#include "tcpip_adapter.h"
/* Initialize Wi-Fi */
static esp_err_t event_handler(void *ctx, system_event_t *event)
{
switch(event->event_id) {
case SYSTEM_EVENT_STA_START:
esp_wifi_connect();
break;
case SYSTEM_EVENT_STA_DISCONNECTED:
esp_wifi_connect();
break;
default:
break;
}
return ESP_OK;
}
void wifi_init_sta(void)
{
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_init(event_handler, NULL));
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
wifi_config_t wifi_config = {
.sta = {
.ssid = "your-ssid",
.password = "your-password",
},
};
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
}
```
2. Create a socket and bind it to a local IP address and port number.
```c
/* Create a TCP server socket */
int server_socket = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP);
if (server_socket == -1) {
ESP_LOGE(TAG, "Failed to create socket: errno %d", errno);
return;
}
/* Bind the socket to a port */
struct sockaddr_in server_address;
server_address.sin_family = AF_INET;
server_address.sin_addr.s_addr = htonl(INADDR_ANY);
server_address.sin_port = htons(80);
int err = bind(server_socket, (struct sockaddr *)&server_address, sizeof(server_address));
if (err != 0) {
ESP_LOGE(TAG, "Socket binding failed: errno %d", errno);
close(server_socket);
return;
}
```
3. Listen for incoming connections.
```c
/* Listen for incoming connections */
err = listen(server_socket, 1);
if (err != 0) {
ESP_LOGE(TAG, "Error occurred while listening for incoming connections: errno %d", errno);
close(server_socket);
return;
}
```
4. Accept incoming connections and handle client requests.
```c
/* Accept incoming connections and handle client requests */
while (1) {
struct sockaddr_in client_address;
socklen_t client_address_len = sizeof(client_address);
int client_socket = accept(server_socket, (struct sockaddr *)&client_address, &client_address_len);
if (client_socket == -1) {
ESP_LOGE(TAG, "Error occurred while accepting incoming connection: errno %d", errno);
continue;
}
char request_buffer[1024] = {0};
read(client_socket, request_buffer, sizeof(request_buffer)-1);
ESP_LOGI(TAG, "Received request: %s", request_buffer);
char response_buffer[1024] = {0};
sprintf(response_buffer, "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<html><body><h1>Hello, World!</h1></body></html>\r\n");
write(client_socket, response_buffer, strlen(response_buffer));
shutdown(client_socket, 0);
close(client_socket);
}
```
In this example, we create a simple HTTP server that responds with "Hello, World!" to any incoming request. You can modify the response to suit your needs.
Note that this is a very basic example and you'll need to add error handling, thread safety, and other features to make it production-ready.
相关推荐
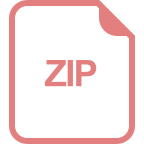














