java查找字符串中次数
时间: 2024-06-04 10:12:47 浏览: 8
您可以使用 Java 中的 String 类的 split() 方法和 length() 方法来实现查找一个字符串中另一个字符串出现的次数。可以按照以下步骤进行操作:
1. 使用 split() 方法把原字符串按照要查找的字符串分割成一个字符串数组
2. 使用 length() 方法获取字符串数组的长度
3. 遍历字符串数组,累加包含要查找字符串的元素的个数即可得到要查找字符串在原字符串中出现的次数。
请注意,这些代码仅供参考,实际查找方法可能因您的具体需求而异。
相关问题
java查找字符串出现的次数
可以使用Java中的String类的方法count来查找一个字符串在另一个字符串中出现的次数。代码示例如下:
```
String str = "hello world, world is beautiful!";
int count = str.count("world");
System.out.println("The word 'world' appears " + count + " times in the string.");
```
输出结果为:
```
The word 'world' appears 2 times in the string.
```
java查找字符串中重复字符
可以使用一个 HashMap 来记录每个字符出现的次数,然后遍历字符串,如果发现某个字符出现次数大于 1,则说明该字符是重复的。以下是 Java 代码示例:
```java
public class DuplicateCharInString {
public static void main(String[] args) {
String str = "Hello, World!";
HashMap<Character, Integer> map = new HashMap<>();
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
if (map.containsKey(c)) {
int count = map.get(c);
map.put(c, count + 1);
} else {
map.put(c, 1);
}
}
for (Map.Entry<Character, Integer> entry : map.entrySet()) {
if (entry.getValue() > 1) {
System.out.println("Duplicate character: " + entry.getKey() + ", count: " + entry.getValue());
}
}
}
}
```
输出结果为:
```
Duplicate character: l, count: 3
Duplicate character: o, count: 2
Duplicate character: !, count: 1
Duplicate character: ,, count: 1
Duplicate character: e, count: 1
Duplicate character: H, count: 1
Duplicate character: W, count: 1
Duplicate character: r, count: 1
```
可以看到,字符串中重复的字符有:l、o。
相关推荐
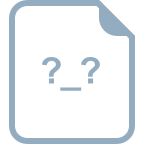












