用c++写一个民航订票系统,使用文件储存数据,不使用链表、容器、数据库,包含用户和管理两个类,实现订票、退票、查询航班、增加航班、删除航班、修改航班、显示航班等功能。
时间: 2023-10-20 07:05:18 浏览: 89
好的,这是一个挑战性比较大的任务,需要耐心和细心地编写代码。下面是一个简单的实现,仅供参考。
```c++
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
class User {
public:
string name;
int age;
string id;
string phone;
User(string name, int age, string id, string phone) {
this->name = name;
this->age = age;
this->id = id;
this->phone = phone;
}
};
class Flight {
public:
string id;
string start;
string end;
string date;
int price;
int seat;
Flight(string id, string start, string end, string date, int price, int seat) {
this->id = id;
this->start = start;
this->end = end;
this->date = date;
this->price = price;
this->seat = seat;
}
};
class Manager {
public:
void addFlight(string id, string start, string end, string date, int price, int seat) {
ofstream outfile("flight.txt", ios::app);
outfile << id << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
outfile.close();
}
void deleteFlight(string id) {
ifstream infile("flight.txt");
ofstream outfile("temp.txt", ios::trunc);
string fid, start, end, date, str;
int price, seat;
while (infile >> fid >> start >> end >> date >> price >> seat) {
if (fid != id) {
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
}
}
infile.close();
outfile.close();
remove("flight.txt");
rename("temp.txt", "flight.txt");
}
void modifyFlight(string id, string start, string end, string date, int price, int seat) {
ifstream infile("flight.txt");
ofstream outfile("temp.txt", ios::trunc);
string fid, s, e, d, str;
int p, s1;
while (infile >> fid >> s >> e >> d >> p >> s1) {
if (fid == id) {
outfile << id << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
} else {
outfile << fid << " " << s << " " << e << " " << d << " " << p << " " << s1 << endl;
}
}
infile.close();
outfile.close();
remove("flight.txt");
rename("temp.txt", "flight.txt");
}
void showFlight() {
ifstream infile("flight.txt");
string id, start, end, date, str;
int price, seat;
while (infile >> id >> start >> end >> date >> price >> seat) {
cout << id << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
}
infile.close();
}
};
class Customer {
public:
User user;
Customer(string name, int age, string id, string phone) {
user = User(name, age, id, phone);
}
void queryFlight(string start, string end, string date) {
ifstream infile("flight.txt");
string id, s, e, d, str;
int p, s1;
while (infile >> id >> s >> e >> d >> p >> s1) {
if (s == start && e == end && d == date && s1 > 0) {
cout << id << " " << start << " " << end << " " << date << " " << p << endl;
}
}
infile.close();
}
void bookFlight(string id) {
ifstream infile("flight.txt");
ofstream outfile("temp.txt", ios::trunc);
string fid, start, end, date, str;
int price, seat;
bool find = false;
while (infile >> fid >> start >> end >> date >> price >> seat) {
if (fid == id) {
if (seat > 0) {
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat - 1 << endl;
find = true;
} else {
cout << "The flight is full." << endl;
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
}
} else {
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
}
}
infile.close();
outfile.close();
if (find) {
remove("flight.txt");
rename("temp.txt", "flight.txt");
} else {
remove("temp.txt");
}
}
void refundFlight(string id) {
ifstream infile("flight.txt");
ofstream outfile("temp.txt", ios::trunc);
string fid, start, end, date, str;
int price, seat;
bool find = false;
while (infile >> fid >> start >> end >> date >> price >> seat) {
if (fid == id) {
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat + 1 << endl;
find = true;
} else {
outfile << fid << " " << start << " " << end << " " << date << " " << price << " " << seat << endl;
}
}
infile.close();
outfile.close();
if (find) {
remove("flight.txt");
rename("temp.txt", "flight.txt");
} else {
remove("temp.txt");
}
}
};
int main() {
Manager manager;
Customer customer("Tom", 20, "123456", "123456789");
string id, start, end, date;
int price, seat;
int choice;
while (true) {
cout << "1. Add flight" << endl;
cout << "2. Delete flight" << endl;
cout << "3. Modify flight" << endl;
cout << "4. Show flight" << endl;
cout << "5. Query flight" << endl;
cout << "6. Book flight" << endl;
cout << "7. Refund flight" << endl;
cout << "0. Quit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Enter the flight id: ";
cin >> id;
cout << "Enter the start city: ";
cin >> start;
cout << "Enter the end city: ";
cin >> end;
cout << "Enter the date: ";
cin >> date;
cout << "Enter the price: ";
cin >> price;
cout << "Enter the seat: ";
cin >> seat;
manager.addFlight(id, start, end, date, price, seat);
break;
case 2:
cout << "Enter the flight id: ";
cin >> id;
manager.deleteFlight(id);
break;
case 3:
cout << "Enter the flight id: ";
cin >> id;
cout << "Enter the start city: ";
cin >> start;
cout << "Enter the end city: ";
cin >> end;
cout << "Enter the date: ";
cin >> date;
cout << "Enter the price: ";
cin >> price;
cout << "Enter the seat: ";
cin >> seat;
manager.modifyFlight(id, start, end, date, price, seat);
break;
case 4:
manager.showFlight();
break;
case 5:
cout << "Enter the start city: ";
cin >> start;
cout << "Enter the end city: ";
cin >> end;
cout << "Enter the date: ";
cin >> date;
customer.queryFlight(start, end, date);
break;
case 6:
cout << "Enter the flight id: ";
cin >> id;
customer.bookFlight(id);
break;
case 7:
cout << "Enter the flight id: ";
cin >> id;
customer.refundFlight(id);
break;
case 0:
exit(0);
default:
cout << "Invalid choice." << endl;
break;
}
}
return 0;
}
```
这个程序使用文件来储存数据,可以对航班进行增加、删除、修改、显示的操作,也可以对航班进行查询、订票、退票的操作。程序中使用了 Manager 和 Customer 两个类来分别实现管理员和顾客的功能。但这个程序还有很多不足之处,比如没有输入校验,没有异常处理等等。
阅读全文
相关推荐
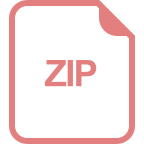
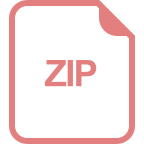
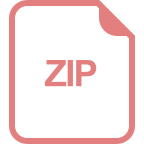
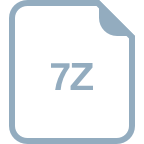
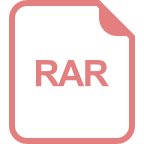
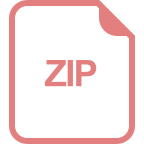
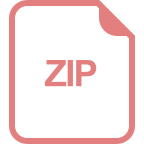
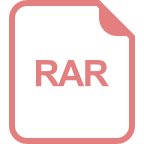
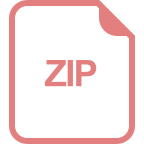
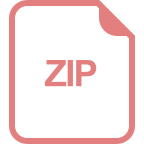
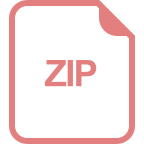
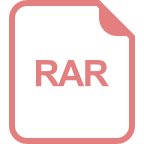