c++在文件score中存有学生的序号、姓名、学号、年龄、性别、总成绩等数据,请编写程序根据指定序号,输出学生数据信息。
时间: 2023-12-22 10:19:33 浏览: 142
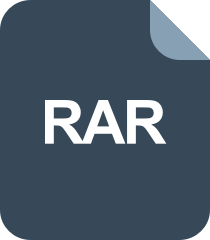
学生成绩查询(c++)
下面是一个简单的C++程序,可以根据指定序号在文件中查找学生数据信息并输出:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
string number;
int age;
string gender;
double score;
};
int main() {
int id;
cout << "请输入要查找的学生序号:";
cin >> id;
ifstream file("score.txt");
if (!file) {
cout << "打开文件失败!" << endl;
return 1;
}
Student student;
bool found = false;
while (file >> student.id >> student.name >> student.number >> student.age >> student.gender >> student.score) {
if (student.id == id) {
found = true;
break;
}
}
if (found) {
cout << "序号:" << student.id << endl;
cout << "姓名:" << student.name << endl;
cout << "学号:" << student.number << endl;
cout << "年龄:" << student.age << endl;
cout << "性别:" << student.gender << endl;
cout << "总成绩:" << student.score << endl;
} else {
cout << "没有找到该学生的数据!" << endl;
}
file.close();
return 0;
}
```
其中,我们定义了一个 `Student` 结构体来表示学生数据。在 `main` 函数中,首先提示用户输入要查找的学生序号,然后打开存储学生数据的文件 `score.txt`。如果文件打开失败,则输出错误信息并退出程序。
接下来,我们定义一个 `Student` 变量 `student`,用来存储从文件中读取的每个学生数据。我们使用一个 `while` 循环,不断从文件中读取数据,直到文件读取结束或者找到指定序号的学生数据为止。如果找到了指定序号的学生数据,则将变量 `found` 标记为 `true`,同时跳出循环。在循环结束后,根据 `found` 变量的值判断是否找到了指定序号的学生数据。如果找到了,则输出该学生的信息,否则输出提示信息。
最后,我们关闭文件并退出程序。
阅读全文
相关推荐
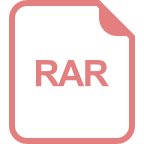
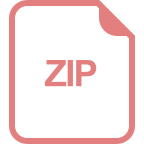
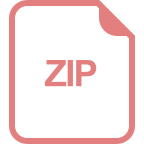
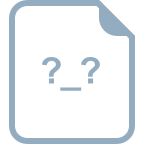
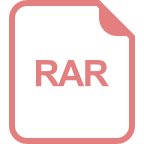
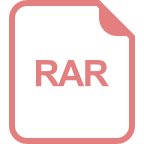
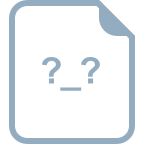
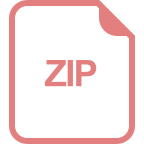
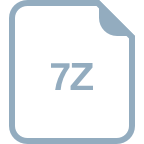
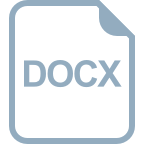
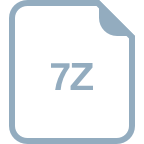