c语言编程有5名学生,每名学生的数据包括学号、姓名、3门课的成绩。从键盘输入5名学生数据,要求输出3门课总平均成绩,以及最高分学生的数据(包括学号、姓名、3门课的成绩、平均分数)
时间: 2023-12-15 08:15:37 浏览: 36
好的,这是一个很好的问题。为了解决这个问题,我们可以采用结构体来存储学生的数据,使用循环语句从键盘输入学生数据,并计算每个学生的平均成绩和3门课总平均成绩,最后输出最高分学生的数据。
以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
#define NUM_STUDENTS 5
#define NUM_COURSES 3
typedef struct {
int id;
char name[20];
float scores[NUM_COURSES];
float avg;
} Student;
int main() {
Student students[NUM_STUDENTS];
float course_avg[NUM_COURSES] = {0};
int max_score_index = 0;
// input data
for (int i = 0; i < NUM_STUDENTS; i++) {
printf("Enter student %d's ID, name and %d course scores: ", i+1, NUM_COURSES);
scanf("%d %s", &students[i].id, students[i].name);
for (int j = 0; j < NUM_COURSES; j++) {
scanf("%f", &students[i].scores[j]);
course_avg[j] += students[i].scores[j];
}
students[i].avg = (students[i].scores[0] + students[i].scores[1] + students[i].scores[2]) / 3.0;
if (students[i].avg > students[max_score_index].avg) {
max_score_index = i;
}
}
// calculate course average
for (int i = 0; i < NUM_COURSES; i++) {
course_avg[i] /= NUM_STUDENTS;
}
// output data
printf("Course average: %.2f %.2f %.2f\n", course_avg[0], course_avg[1], course_avg[2]);
printf("Student with highest score:\n");
printf("ID: %d\n", students[max_score_index].id);
printf("Name: %s\n", students[max_score_index].name);
printf("Scores: %.2f %.2f %.2f\n", students[max_score_index].scores[0], students[max_score_index].scores[1], students[max_score_index].scores[2]);
printf("Average score: %.2f\n", students[max_score_index].avg);
return 0;
}
```
这个程序会要求输入每个学生的ID、姓名和3门课的成绩,然后计算每个学生的平均成绩和3门课总平均成绩,最后输出最高分学生的数据。注意,这个程序假设每门课的满分是100分。
相关推荐
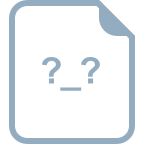
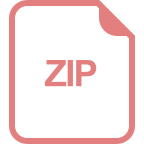
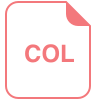
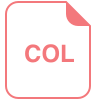
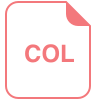
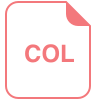
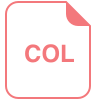









