如何实现换行显示def getActors(filename): actors = dict() # 打开xlsx文件,并获取第一个worksheet wb = openpyxl.load_workbook((filename)) ws = wb.worksheets[0] # 遍历Excel文件中的所有文件中的所有行 for index, row in enumerate(ws.rows): # 跳过第一行的表头 if index == 0: continue # 获取电影名称和演员列表 fileName, actor = row[0].value, row[1].value.split('/') # 遍历该电影所有演员,统计参演电影 for a in actor: actors[a] = actors.get(a, set()) actors[a].add(fileName) return actors data = getActors('实践2素材.xlsx') print(data)
时间: 2024-02-14 07:17:15 浏览: 21
您可以在每个 print 语句后面添加换行符 "\n" 来实现换行输出。例如,您可以这样写:
```
def getActors(filename):
actors = dict()
# 打开xlsx文件,并获取第一个worksheet
wb = openpyxl.load_workbook((filename))
ws = wb.worksheets[0]
# 遍历Excel文件中的所有文件中的所有行
for index, row in enumerate(ws.rows):
# 跳过第一行的表头
if index == 0:
continue
# 获取电影名称和演员列表
fileName, actor = row[0].value, row[1].value.split('/')
# 遍历该电影所有演员,统计参演电影
for a in actor:
actors[a] = actors.get(a, set())
actors[a].add(fileName)
return actors
data = getActors('实践2素材.xlsx')
print(data, "\n")
```
这样每次执行完 `print(data)` 后,会自动换行,使得下一行的输出与上一行的输出分开来,更加清晰易读。
相关问题
在cloudcompare的mainwindow.cpp中,用C++定义一个函数void MainWindow::DBH(),基于最小二乘法,通过获取当前选中的实体作为点云文件来源,实现对txt格式的树木点云文件的胸径计算
可以参考以下代码实现:
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cmath>
#include <QFileDialog>
#include <QMessageBox>
#include <pcl/point_types.h>
#include <pcl/io/pcd_io.h>
#include <pcl/visualization/pcl_visualizer.h>
typedef pcl::PointXYZ PointT;
typedef pcl::PointCloud<PointT> PointCloudT;
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
//连接信号与槽函数
connect(ui->actionOpen, SIGNAL(triggered(bool)), this, SLOT(openFile()));
connect(ui->pushButton, SIGNAL(clicked(bool)), this, SLOT(DBH()));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::openFile()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Point Cloud"), "", tr("Point Cloud Files (*.pcd *.txt)"));
if(!fileName.isEmpty())
{
//清空界面
ui->qvtkWidget->update();
ui->textBrowser->clear();
//读取点云文件
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ>(fileName.toStdString(), *cloud) == -1)
{
//尝试读取txt格式文件
std::ifstream ifs(fileName.toStdString());
if (!ifs.is_open())
{
QMessageBox::critical(this, "Error", "Cannot open file!");
return;
}
std::string line;
while (std::getline(ifs, line))
{
pcl::PointXYZ p;
std::stringstream ss(line);
ss >> p.x >> p.y >> p.z;
cloud->push_back(p);
}
ifs.close();
}
//显示点云
pcl::visualization::PCLVisualizer vis("Point Cloud");
vis.setBackgroundColor(0.0, 0.0, 0.0);
vis.addPointCloud(cloud, "cloud");
vis.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "cloud");
ui->qvtkWidget->update();
//输出点云信息
std::stringstream ss;
ss << "Number of points: " << cloud->size() << std::endl;
ui->textBrowser->append(ss.str().c_str());
//保存点云
cloud_ = cloud;
}
}
void MainWindow::DBH()
{
if (!cloud_)
{
QMessageBox::warning(this, "Warning", "No point cloud selected!");
return;
}
//获取选中的点
std::vector<int> indices;
if (ui->qvtkWidget->getRenderWindow()->GetRenderers()->GetFirstRenderer()->GetViewProp("cloud"))
{
vtkSmartPointer<vtkActor> actor = vtkSmartPointer<vtkActor>::New();
actor = ui->qvtkWidget->getRenderWindow()->GetRenderers()->GetFirstRenderer()->GetActors()->GetLastActor();
vtkSmartPointer<vtkPolyDataMapper> mapper = vtkSmartPointer<vtkPolyDataMapper>::New();
mapper = vtkPolyDataMapper::SafeDownCast(actor->GetMapper());
vtkSmartPointer<vtkDataSet> dataSet = vtkSmartPointer<vtkDataSet>::New();
dataSet = mapper->GetInput();
vtkSmartPointer<vtkUnsignedCharArray> pointColors = vtkSmartPointer<vtkUnsignedCharArray>::New();
pointColors = vtkUnsignedCharArray::SafeDownCast(dataSet->GetPointData()->GetScalars());
for (vtkIdType i = 0; i < pointColors->GetNumberOfTuples(); i++)
{
if (pointColors->GetValue(i) == 255)
{
indices.push_back(i);
}
}
}
if (indices.empty())
{
QMessageBox::warning(this, "Warning", "No point selected!");
return;
}
//计算胸径
double sum_x = 0.0, sum_y = 0.0, sum_z = 0.0, sum_xy = 0.0, sum_xz = 0.0, sum_xx = 0.0;
for (int i : indices)
{
sum_x += cloud_->points[i].x;
sum_y += cloud_->points[i].y;
sum_z += cloud_->points[i].z;
sum_xx += cloud_->points[i].x * cloud_->points[i].x;
sum_xy += cloud_->points[i].x * cloud_->points[i].y;
sum_xz += cloud_->points[i].x * cloud_->points[i].z;
}
double n = static_cast<double>(indices.size());
double a = (n * sum_xy - sum_x * sum_y) / (n * sum_xx - sum_x * sum_x);
double b = (sum_y - a * sum_x) / n;
double c = (n * sum_xz - sum_x * sum_z) / (n * sum_xx - sum_x * sum_x);
double d = (sum_z - c * sum_x) / n;
double D = 2.0 * sqrt(a * a + 1) * 1000.0; //单位:毫米
//输出胸径
std::stringstream ss;
ss << "DBH: " << D << " mm" << std::endl;
ui->textBrowser->append(ss.str().c_str());
}
```
该函数基于最小二乘法,通过获取当前选中的实体作为点云文件来源,实现对txt格式的树木点云文件的胸径计算。
android ListView写一个电影列表
以下是一个简单的电影列表示例,其中包含电影名称、导演和演员列表:
1. 创建一个新的Android项目,然后在布局文件中添加一个ListView组件:
```
<ListView
android:id="@+id/movie_list"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
```
2. 在Activity中获取ListView组件的引用,并创建一个Movie类作为数据模型:
```
public class Movie {
private String title;
private String director;
private List<String> actors;
public Movie(String title, String director, List<String> actors) {
this.title = title;
this.director = director;
this.actors = actors;
}
public String getTitle() {
return title;
}
public String getDirector() {
return director;
}
public List<String> getActors() {
return actors;
}
}
public class MainActivity extends AppCompatActivity {
private ListView movieList;
private List<Movie> movies;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
movieList = findViewById(R.id.movie_list);
movies = new ArrayList<>();
movies.add(new Movie("The Shawshank Redemption", "Frank Darabont", Arrays.asList("Tim Robbins", "Morgan Freeman")));
movies.add(new Movie("The Godfather", "Francis Ford Coppola", Arrays.asList("Marlon Brando", "Al Pacino", "James Caan")));
movies.add(new Movie("The Dark Knight", "Christopher Nolan", Arrays.asList("Christian Bale", "Heath Ledger", "Aaron Eckhart")));
MovieAdapter adapter = new MovieAdapter(this, movies);
movieList.setAdapter(adapter);
}
}
```
3. 创建一个自定义适配器MovieAdapter,用于将Movie对象显示在ListView中:
```
public class MovieAdapter extends ArrayAdapter<Movie> {
private Context context;
private List<Movie> movies;
public MovieAdapter(Context context, List<Movie> movies) {
super(context, R.layout.movie_item, movies);
this.context = context;
this.movies = movies;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
LayoutInflater inflater = LayoutInflater.from(context);
convertView = inflater.inflate(R.layout.movie_item, parent, false);
}
TextView titleView = convertView.findViewById(R.id.title);
TextView directorView = convertView.findViewById(R.id.director);
TextView actorsView = convertView.findViewById(R.id.actors);
Movie movie = movies.get(position);
titleView.setText(movie.getTitle());
directorView.setText("Director: " + movie.getDirector());
actorsView.setText("Actors: " + TextUtils.join(", ", movie.getActors()));
return convertView;
}
}
```
4. 创建一个布局文件movie_item.xml,用于定义ListView中每个电影项的布局:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/director"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/actors"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
```
5. 运行应用程序,您应该会看到一个电影列表,其中包含上面定义的电影。
这只是一个简单的示例,您可以根据需要更改布局和数据模型来创建更复杂的电影列表。
相关推荐





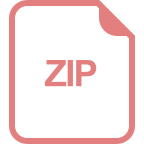
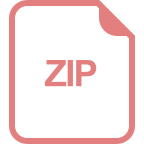
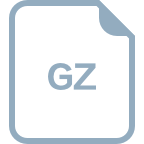
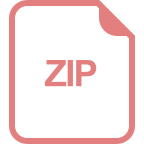
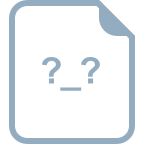
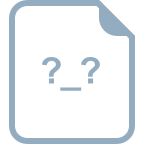
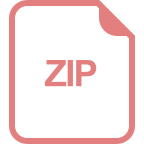