springboot mybatis根据输入的日期查询数据条数
时间: 2023-12-19 19:07:12 浏览: 23
假设有一个MyBatis的Mapper类和对应的XML文件,可以使用以下方式来根据输入的日期查询数据条数:
Mapper类:
```
public interface MyMapper {
int getCountByDate(@Param("date") Date date);
}
```
XML文件:
```
<select id="getCountByDate" resultType="int">
SELECT COUNT(*) FROM my_table
WHERE date_column = #{date}
</select>
```
然后在Spring Boot的Service或Controller中注入MyMapper,并调用getCountByDate方法即可:
```
@Service
public class MyService {
@Autowired
private MyMapper myMapper;
public int getCountByDate(Date date) {
return myMapper.getCountByDate(date);
}
}
```
相关问题
springboot mybatis根据输入的日期查询数据条数在前端显示
假设你有一个MyBatis Mapper接口和对应的XML文件,其中有一个查询方法,可以根据日期查询数据条数。你可以在Spring Boot中创建一个Controller来处理前端请求,并调用该方法来获取数据条数。
首先,在你的Controller中注入你的Mapper接口:
```
@Autowired
private YourMapper yourMapper;
```
然后,创建一个RequestMapping方法来处理前端请求:
```
@RequestMapping("/countByDate")
public String getCountByDate(Model model, @RequestParam("date") String date) {
int count = yourMapper.getCountByDate(date);
model.addAttribute("count", count);
return "countByDate";
}
```
这个方法将接受一个名为“date”的请求参数,并使用它调用你的Mapper接口中的“getCountByDate”方法来获取数据条数。然后,将数据条数添加到一个名为“count”的Model属性中,并将其返回到名为“countByDate”的视图中。
最后,在你的视图中使用EL表达式来显示数据条数:
```
<p>There are ${count} records for the selected date.</p>
```
这是一个非常简单的示例,你可以根据你的具体需求进行调整。
springboot mybatis 千万级数据查询
为了在SpringBoot Mybatis中高效地查询千万级数据,可以采用以下几种方法:
1.使用分页查询:可以使用Mybatis的分页插件PageHelper来实现分页查询,将大量数据分成多个小块进行查询,提高查询效率。
```java
//引入PageHelper插件
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.13</version>
</dependency>
//在Mapper接口中使用分页查询
@Select("select * from table_name")
List<Table> selectByPage(@Param("pageNum") int pageNum, @Param("pageSize") int pageSize);
```
2.使用缓存:可以使用Mybatis的二级缓存来缓存查询结果,避免重复查询,提高查询效率。
```java
//在Mybatis配置文件中开启二级缓存
<settings>
<setting name="cacheEnabled" value="true"/>
</settings>
//在Mapper接口中使用缓存查询
@Select("select * from table_name")
@Cacheable(cacheNames = "tableCache")
List<Table> selectAll();
```
3.使用索引:可以在查询的字段上创建索引,加快查询速度。
```sql
//在查询的字段上创建索引
CREATE INDEX index_name ON table_name(column_name);
```
相关推荐
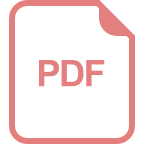
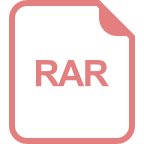












