java完成登陆界面的设计,并能点击✕关闭窗口。在窗口最上方设置一文本行,不与用户名和密码在文本行重叠,设计用户名为herry,密码为则12456,输入用户名和密码后点击OK如果和你设计的用户名和密码相同则在窗口最上面设置的文本行中显示登陆成功,否则显示登陆失败。点击Cancel,则把文本行置空。
时间: 2024-01-21 14:15:57 浏览: 18
以下是一个简单的Java登陆界面的设计:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LoginFrame extends JFrame implements ActionListener {
JLabel userLabel, passwordLabel, messageLabel;
JTextField userField, passwordField;
JButton okButton, cancelButton;
public LoginFrame() {
setLayout(new GridLayout(3, 2));
userLabel = new JLabel("用户名:");
add(userLabel);
userField = new JTextField();
add(userField);
passwordLabel = new JLabel("密码:");
add(passwordLabel);
passwordField = new JPasswordField();
add(passwordField);
okButton = new JButton("OK");
okButton.addActionListener(this);
add(okButton);
cancelButton = new JButton("Cancel");
cancelButton.addActionListener(this);
add(cancelButton);
messageLabel = new JLabel();
messageLabel.setHorizontalAlignment(JLabel.CENTER);
add(messageLabel);
setTitle("登陆界面");
setSize(300, 150);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new LoginFrame();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == okButton) {
String user = userField.getText();
String password = passwordField.getText();
if (user.equals("herry") && password.equals("12456")) {
messageLabel.setText("登陆成功");
} else {
messageLabel.setText("登陆失败");
}
} else if (e.getSource() == cancelButton) {
messageLabel.setText("");
userField.setText("");
passwordField.setText("");
}
}
}
```
在这个登陆界面中,我们使用了`JLabel`、`JTextField`、`JPasswordField`、`JButton`和`GridLayout`等Swing组件和布局。
其中,`JLabel`用于显示文本,`JTextField`和`JPasswordField`用于输入文本,`JButton`用于触发事件,`GridLayout`用于设置组件的布局。
在`actionPerformed`方法中,我们判断点击的按钮是OK还是Cancel,分别执行不同的代码逻辑。如果点击的是OK按钮,我们获取用户名和密码,判断是否与预设的值相同,如果相同则在文本行中显示“登陆成功”,否则显示“登陆失败”;如果点击的是Cancel按钮,我们把文本行、用户名和密码都置空。
此外,我们还通过调用`setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)`方法来设置窗口关闭时退出程序,调用`setVisible(true)`方法来显示窗口。
相关推荐
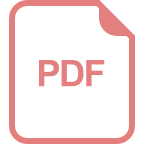
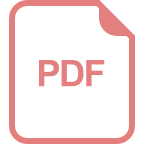













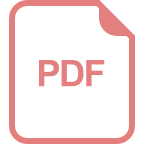
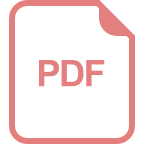