用c语言编写一个具有进栈出栈操作的链式栈
时间: 2024-05-15 07:17:21 浏览: 81
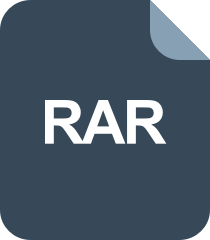
shunxubiao.rar_栈的顺序表_顺序栈,出栈
好的,可以给您提供参考代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct stack {
Node *top;
} Stack;
void init(Stack *stack) {
stack->top = NULL;
}
int isEmpty(Stack *stack) {
return stack->top == NULL;
}
void push(Stack *stack, int data) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = stack->top;
stack->top = newNode;
}
int pop(Stack *stack) {
if (isEmpty(stack)) {
printf("Error: stack is empty\n");
return -1;
}
int data = stack->top->data;
Node *temp = stack->top;
stack->top = stack->top->next;
free(temp);
return data;
}
void print(Stack *stack) {
if (isEmpty(stack)) {
printf("Stack is empty\n");
return;
}
Node *temp = stack->top;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
Stack stack;
init(&stack);
push(&stack, 1);
push(&stack, 2);
push(&stack, 3);
print(&stack);
int data = pop(&stack);
printf("pop: %d\n", data);
print(&stack);
return 0;
}
```
阅读全文
相关推荐
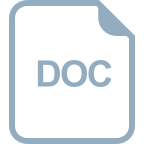
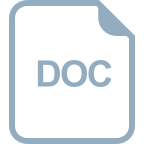

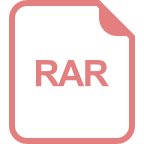
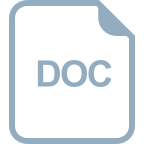


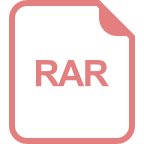
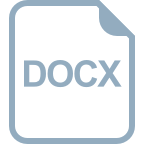
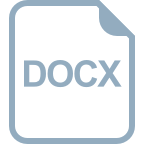
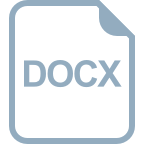
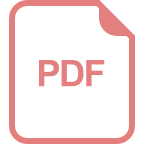
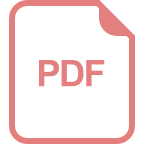
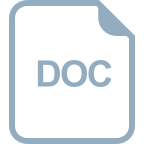
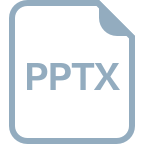
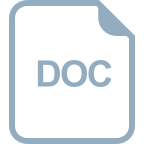
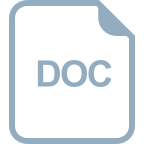
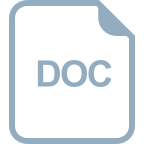