使用 tkinter 编写python程序,实现如下图所示的电子时钟。 要求: 不显示标题栏,总是顶端显示,不被其他窗口覆盖; 实时显示日期和时间; 可以用鼠标左键按住拖动,在电子时钟上右击可以结束程序; 拖动时透明度变大,鼠标左键抬起时恢复半透明状态
时间: 2024-05-12 17:18:54 浏览: 87
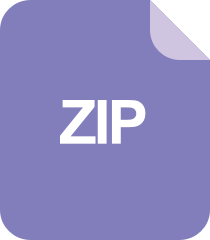
用代码实现电子时钟
这里提供一份基本的代码,实现了上述要求:
```python
import tkinter as tk
from datetime import datetime
class Clock:
def __init__(self):
self.window = tk.Tk()
self.window.overrideredirect(True)
self.window.attributes('-topmost', True)
self.window.bind('<Button-1>', self.start_move)
self.window.bind('<B1-Motion>', self.on_move)
self.window.bind('<ButtonRelease-1>', self.stop_move)
self.window.bind('<Button-3>', self.quit)
self.label = tk.Label(self.window, font=('Arial', 80), fg='white', bg='black')
self.label.pack(expand=True, fill=tk.BOTH)
self.window.geometry('400x200')
self.window.attributes('-alpha', 0.5)
self.window.mainloop()
def start_move(self, event):
self.x, self.y = event.x, event.y
self.window.attributes('-alpha', 1)
def on_move(self, event):
deltax = event.x - self.x
deltay = event.y - self.y
x = self.window.winfo_x() + deltax
y = self.window.winfo_y() + deltay
self.window.geometry(f'+{x}+{y}')
def stop_move(self, event):
self.window.attributes('-alpha', 0.5)
def quit(self, event):
self.window.destroy()
def update_time(self):
now = datetime.now()
self.label.config(text=now.strftime('%Y-%m-%d %H:%M:%S'))
self.window.after(1000, self.update_time)
def run(self):
self.update_time()
self.window.mainloop()
if __name__ == '__main__':
clock = Clock()
clock.run()
```
这个程序实现了一个简单的时钟,可以拖动窗口位置,右键结束程序,显示半透明的窗口。在 `update_time` 方法中实时更新时间,使用 `after` 方法定时执行。
阅读全文
相关推荐
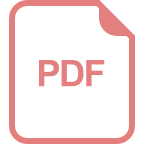
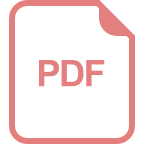
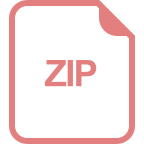
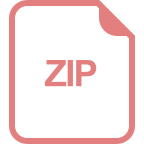
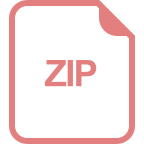
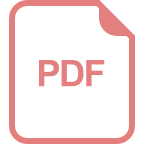
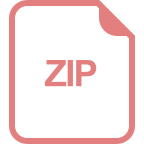
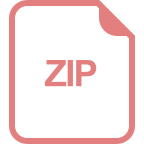
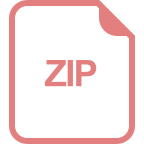
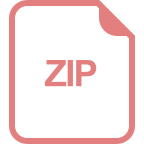
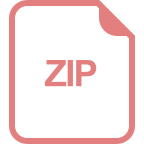
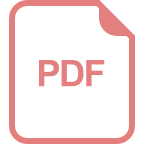
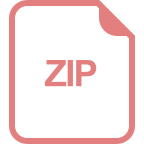
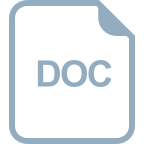
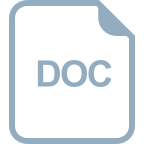
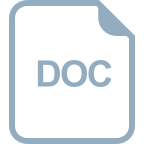
