用python实现推箱子小游戏并伴有多个关卡
时间: 2023-09-17 22:07:23 浏览: 95
好的,推箱子小游戏可以使用Pygame库来实现,可以使用多个关卡的方式是在每个关卡的地图文件中定义不同的地图,然后在游戏中切换关卡时载入不同的地图文件。
以下是一个简单的推箱子小游戏代码示例:
```python
import pygame
import sys
# 游戏窗口大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# 箱子和目的地的大小
BOX_SIZE = 40
GOAL_SIZE = 40
# 地图数据
maps = [
[
"##########",
"# #",
"# #",
"# #",
"# o ### #",
"# o # #",
"# o # # #",
"# ## #",
"######## #",
" #",
" #",
" #",
" #",
" #",
" #",
" #",
"###########",
],
[
"##########",
"# #",
"# #",
"# #",
"# #",
"# o ### #",
"# o #",
"# o # #",
"# ## #",
"# o #",
"# o #",
"# o #",
"# #",
"# #",
"# #",
"# #",
"###########",
]
]
# 地图数据转化为图像
def draw_map(map_data):
box_img = pygame.Surface((BOX_SIZE, BOX_SIZE))
box_img.fill((255, 255, 0))
goal_img = pygame.Surface((GOAL_SIZE, GOAL_SIZE))
goal_img.fill((0, 255, 0))
wall_img = pygame.Surface((BOX_SIZE, BOX_SIZE))
wall_img.fill((0, 0, 255))
bg_img = pygame.Surface((SCREEN_WIDTH, SCREEN_HEIGHT))
bg_img.fill((255, 255, 255))
x = y = 0
for row in map_data:
for col in row:
if col == "#":
screen.blit(wall_img, (x, y))
elif col == "o":
screen.blit(box_img, (x, y))
elif col == "*":
screen.blit(goal_img, (x, y))
x += BOX_SIZE
y += BOX_SIZE
x = 0
screen.blit(bg_img, (0, 0))
# 移动箱子
def move_box(x, y, dx, dy, map_data):
if map_data[y+dy][x+dx] not in ("#", "o"):
map_data[y][x] = " "
map_data[y+dy][x+dx] = "o"
return True
return False
# 判断是否胜利
def is_win(map_data):
for row in map_data:
if "o" in row:
return False
return True
pygame.init()
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("推箱子小游戏")
clock = pygame.time.Clock()
map_index = 0
map_data = maps[map_index]
draw_map(map_data)
while True:
clock.tick(30)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
elif event.key == pygame.K_r:
map_data = maps[map_index]
draw_map(map_data)
elif event.key == pygame.K_LEFT:
for y, row in enumerate(map_data):
if "o" in row:
x = row.index("o")
if move_box(x, y, -1, 0, map_data):
draw_map(map_data)
break
elif event.key == pygame.K_RIGHT:
for y, row in enumerate(map_data):
if "o" in row:
x = row.index("o")
if move_box(x, y, 1, 0, map_data):
draw_map(map_data)
break
elif event.key == pygame.K_UP:
for y, row in enumerate(map_data):
if "o" in row:
x = row.index("o")
if move_box(x, y, 0, -1, map_data):
draw_map(map_data)
break
elif event.key == pygame.K_DOWN:
for y, row in enumerate(map_data):
if "o" in row:
x = row.index("o")
if move_box(x, y, 0, 1, map_data):
draw_map(map_data)
break
elif event.key == pygame.K_n:
map_index += 1
if map_index >= len(maps):
map_index = 0
map_data = maps[map_index]
draw_map(map_data)
if is_win(map_data):
map_index += 1
if map_index >= len(maps):
map_index = 0
map_data = maps[map_index]
draw_map(map_data)
pygame.display.update()
```
这个示例代码中只包含了两个地图,你可以自己定义更多的地图,并将它们存储在地图文件中,然后在代码中加载它们。
相关推荐
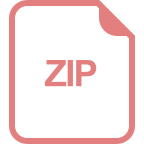
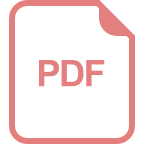
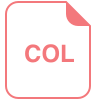
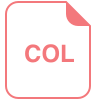
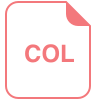
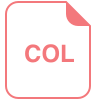
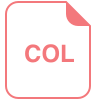









