#include <iostream> using namespace std; int count = 0; class Point { int x, y; public: Point() { x = 1; y = 1; count++; } ~Point() { count--; } friend void play(); }; void play() { count++; } void main() { Point a; play(); { Point b[5]; play(); } play(); cout << count << endl; }输出结果
时间: 2024-01-13 17:02:15 浏览: 102
这段代码中,主函数的返回值类型应该是int而不是void,因此需要将 void main() 改为 int main()。
修改后的代码:
```cpp
#include <iostream>
using namespace std;
int count = 0;
class Point {
int x, y;
public:
Point() {
x = 1; y = 1;
count++;
}
~Point() {
count--;
}
friend void play();
};
void play() {
count++;
}
int main() {
Point a;
play();
{
Point b[5];
play();
}
play();
cout << count << endl;
return 0;
}
```
输出结果是:
```
8
```
程序中创建了一个 Point 对象 a,一个 Point 类型的数组 b,数组中有 5 个元素。在 main 函数中调用了 play 函数三次,让 count 加 3。因此,在 b 数组创建时,会调用 5 次 Point 的构造函数,让 count 加 5。在 b 数组销毁时,会调用 5 次 Point 的析构函数,让 count 减 5。因此,最终输出的 count 的值是 8。
相关问题
以下程序执行后为什么sp3的引用计数为2?#include <memory> #include <iostream> using namespace std; class Point : public std::enable_shared_from_this<Point> { public: Point(int ix = 0, int iy = 0) : _ix(ix) , _iy(iy) { cout << "Point(int,int)" << endl; } ~Point() { cout << "~Point()" << endl; } shared_ptr<Point> addPoint(Point & pt) { _ix += pt._ix; _iy += pt._iy; return shared_from_this(); } private: int _ix; int _iy; }; void test() { shared_ptr<Point> sp1(new Point(1, 2)); shared_ptr<Point> sp2(new Point(11, 12)); shared_ptr<Point> sp3(sp1->addPoint(*sp2)); cout << "sp1.use_count = " << sp1.use_count() << endl; cout << "sp2.use_count = " << sp2.use_count() << endl; cout << "sp3.use_count = " << sp3.use_count() << endl; } int main() { test(); return 0; }
这是因为在类 Point 中使用了 std::enable_shared_from_this,它是一个模板类,可以让一个对象 shared_ptr 类型的智能指针来管理。在类 Point 中,addPoint 函数返回的是 shared_from_this(),它会返回一个指向此对象的 shared_ptr 智能指针,这个指针会增加对象的引用计数,因此 sp3 的引用计数为2。如果没有使用 std::enable_shared_from_this,addPoint 函数返回的是一个普通指针,此时 sp3 的引用计数就是1。
#include <iostream>#include <cmath>using namespace std;class Point {private: int x; int y; static int count; // 统计点的个数public: Point(int x = 0, int y = 0) { this->x = x; this->y = y; count++; } int getX() { return x; } int getY() { return y; } void display() { cout << "x: " << x << ", y: " << y << endl; } Point midpoint(int x, int y) const { int midX = (this->x + x) / 2; int midY = (this->y + y) / 2; return Point(midX, midY); } static void showCount() { cout << "The count of points is: " << count << endl; } friend double distance(Point p); // 求出点到原点的距离};int Point::count = 0;double distance(Point p) { return sqrt(pow(p.x, 2) + pow(p.y, 2));}int main() { Point p1(1, 2); Point p2(3, 4); Point::showCount(); // 输出点的个数 p1.display(); // 输出点的坐标 p2.display(); cout << "The distance between p1 and origin is: " << distance(p1) << endl; // 输出点到原点的距离 Point mid = p1.midpoint(3, 4); // 计算点与原点之间的中点 mid.display(); const Point* p = &p2; // 常指针 p->getX(); // 用常指针调用getX函数 p->getY(); // 用常指针调用getY函数 p->midpoint(5, 6); // 用常指针调用midpoint函数,因为midpoint函数是常函数,所以可以用常指针调用 //p->display(); // 错误,display函数不是常函数,不能用常指针调用 return 0;}结果说明
以上代码是一个简单的面向对象程序,定义了一个Point类,表示一个二维平面上的点,并实现了一些基本的点操作,包括输出点的坐标、计算点之间的中点、计算点到原点的距离等。同时,还定义了一个静态成员变量count,用于统计点的个数,以及一个友元函数distance,用于求出点到原点的距离。
在main函数中,通过创建Point对象,调用类的成员函数以及静态成员函数,展示了Point类的基本使用方法。同时,还通过常指针演示了如何使用常函数以及不能使用常指针调用非常函数的情况。程序最后输出了点的个数、点的坐标、点到原点的距离以及点与原点之间的中点坐标。
阅读全文
相关推荐
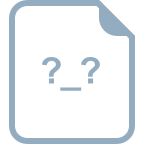
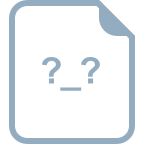

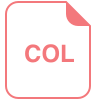
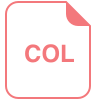
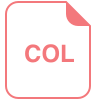
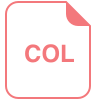
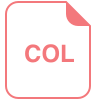
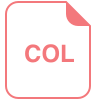
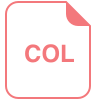
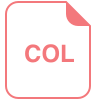
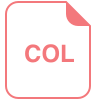
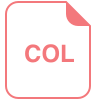
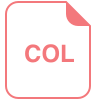

