vue录音功能
时间: 2023-06-29 08:05:31 浏览: 163
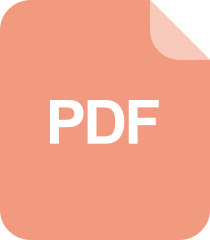
vue使用recorder.js实现录音功能

要在Vue中实现录音功能,可以考虑使用Web API中的MediaRecorder和getUserMedia方法。这些API允许您访问用户的麦克风并将音频数据录制到文件中。
以下是一个简单的Vue示例,演示如何实现录音功能:
1.首先,您需要在Vue组件中引入MediaRecorder和getUserMedia:
```javascript
import { getUserMedia } from 'webrtc-adapter';
import MediaRecorder from 'audio-recorder-polyfill';
```
2.在Vue组件的data属性中添加一个名为“recording”的布尔值,用于跟踪录音状态:
```javascript
data() {
return {
recording: false
}
}
```
3.在Vue组件的methods属性中添加一个名为“startRecording”的方法,用于开始录音:
```javascript
methods: {
startRecording() {
this.recording = true;
const constraints = { audio: true };
navigator.mediaDevices.getUserMedia(constraints)
.then(stream => {
const options = { mimeType: 'audio/webm' };
const mediaRecorder = new MediaRecorder(stream, options);
mediaRecorder.start();
mediaRecorder.ondataavailable = event => {
const audioFile = new File([event.data], 'recording.webm');
// Do something with the audio file, e.g. upload it to a server
};
this.mediaRecorder = mediaRecorder;
})
.catch(error => {
console.error('Error accessing user media:', error);
});
}
}
```
4.在Vue组件的template中添加一个按钮来启动录音:
```html
<template>
<div>
<button v-if="!recording" @click="startRecording">Start Recording</button>
<button v-else @click="stopRecording">Stop Recording</button>
</div>
</template>
```
5.添加一个名为“stopRecording”的方法,用于停止录音:
```javascript
stopRecording() {
this.recording = false;
this.mediaRecorder.stop();
}
```
这就是如何在Vue中实现录音功能的简单示例。请注意,此示例中使用的MediaRecorder和getUserMedia API可能需要polyfill来确保与所有浏览器兼容。
阅读全文
相关推荐
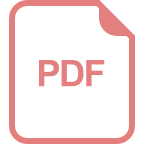
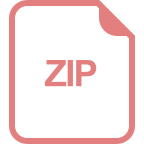
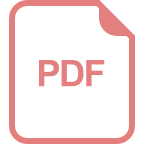
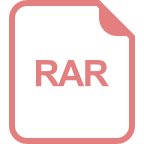
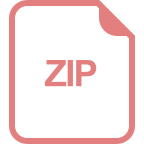
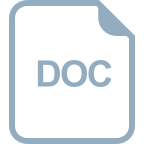
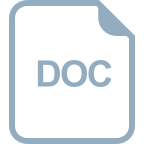
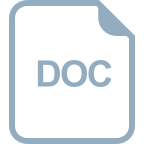



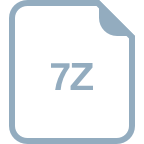
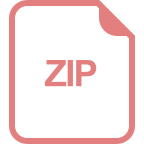


