将这个json文件动态加载入HTML的option中。json文件{ "problem_categories": [ { "name": "产品质量问题", "id": 1, "description": "客户反映产品质量有问题,无法正常使用或有明显缺陷" }, { "name": "服务态度问题", "id": 2, "description": "客户投诉服务人员态度不友好或存在其他不专业问题" }, { "name": "交付时间问题", "id": 3, "description": "客户反馈交付时间不准确或延迟,影响下一步工作计划" }, { "name": "其他", "id": 4, "description": "客户反馈的问题不属于以上三种类型" } ] }
时间: 2023-05-24 11:05:20 浏览: 100
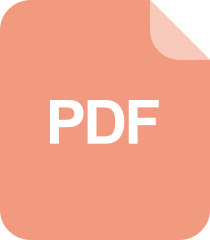
vue.js学习笔记:如何加载本地json文件

<!DOCTYPE html>
<html>
<head>
<title>Dynamic Dropdown from JSON</title>
</head>
<body>
<label for="category">Problem Category: </label>
<select id="category">
<option value="">Select a category</option>
</select>
<script>
const selectCategory = document.querySelector('#category');
fetch('problem_categories.json')
.then(response => response.json())
.then(json => {
json.problem_categories.forEach(category => {
const option = document.createElement('option');
option.value = category.id;
option.textContent = category.name;
selectCategory.appendChild(option);
});
})
.catch(error => console.log(error));
</script>
</body>
</html>
阅读全文
相关推荐
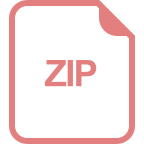
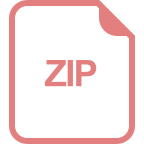








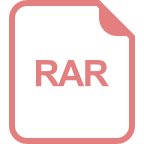
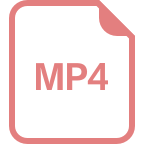
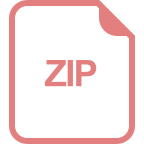
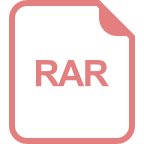