python日记本程序要求 1. 具有新建、打开、关闭、保存、另存日记本等文件操作功能; 2. 提供日记编辑功能,每篇日记包括三部分:标题、内容及附加信息,保存时这三部分信息全部保存 A. 标题:普通文本输入; B. 内容:富文本形式的编辑器,能够编辑图文(要求能够插入图片)日记内容; C. 附加信息:日记编辑界面某个特定位置(附加信息显示区)显示当前日期时间、天气情况、根据IP获取的地理定位信息。 3. 可以为日记本设定密码,设定了密码的日记本在打开时提示输入密码,只有输入了正确密码后才可以打开; 密码
时间: 2024-03-17 08:46:52 浏览: 184
保护功能可以通过使用Python内置的加密模块来实现。以下是一个简单的示例代码,可以实现对日记本的加密和解密:
```python
from cryptography.fernet import Fernet
class Diary:
def __init__(self, password=None):
self.password = password
self.title = ''
self.content = ''
self.additional_info = ''
self.file_path = ''
def set_password(self, password):
self.password = password
def save(self):
if self.password:
key = Fernet.generate_key()
cipher_suite = Fernet(key)
self.title = cipher_suite.encrypt(self.title.encode())
self.content = cipher_suite.encrypt(self.content.encode())
self.additional_info = cipher_suite.encrypt(self.additional_info.encode())
with open(self.file_path, 'wb') as f:
f.write(key)
f.write(self.title)
f.write(self.content)
f.write(self.additional_info)
else:
with open(self.file_path, 'w') as f:
f.write(self.title)
f.write(self.content)
f.write(self.additional_info)
def open(self):
if self.password:
with open(self.file_path, 'rb') as f:
key = f.read(32)
cipher_suite = Fernet(key)
self.title = cipher_suite.decrypt(f.read())
self.content = cipher_suite.decrypt(f.read())
self.additional_info = cipher_suite.decrypt(f.read())
else:
with open(self.file_path, 'r') as f:
self.title = f.readline()
self.content = f.readline()
self.additional_info = f.readline()
def close(self):
self.title = ''
self.content = ''
self.additional_info = ''
def set_file_path(self, file_path):
self.file_path = file_path
```
在这个示例代码中,我们使用了`cryptography`模块中的`Fernet`进行加密和解密操作。如果`Diary`对象的`password`属性不为空,则在调用`save()`方法时会对`title`、`content`和`additional_info`进行加密,并将加密后的数据和密钥写入文件中。在调用`open()`方法时,会先读取密钥,然后使用密钥对文件中的数据进行解密。这样,即使别人获得了日记本文件,也无法直接查看其中的内容。
当然,这只是一个简单的示例代码,还有很多可以改进的地方,例如可以使用更加安全的加密算法、增加密码输入错误次数限制等等。
阅读全文
相关推荐







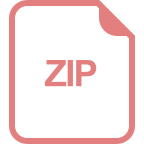