springboot后端将文件封装为对象传到前端接收
时间: 2024-05-15 10:16:14 浏览: 105
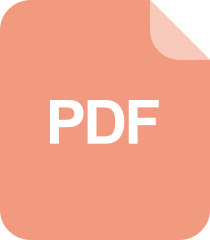
springboot如何去获取前端传递的参数的实现
在Spring Boot后端中,可以使用Java的File类来操作文件,读取文件内容并将其封装为对象传递到前端。
首先,需要定义一个Java类来表示文件对象,可以包含文件名、文件路径、文件内容等属性。例如:
```
public class FileObject {
private String fileName;
private String filePath;
private String fileContent;
// getter and setter methods
}
```
然后,在后端中使用File类来读取文件内容,并将其封装为FileObject对象。可以使用以下代码:
```
File file = new File(filePath); // filePath为文件路径
String fileContent = new String(Files.readAllBytes(file.toPath()), StandardCharsets.UTF_8); // 读取文件内容
FileObject fileObject = new FileObject();
fileObject.setFileName(file.getName());
fileObject.setFilePath(filePath);
fileObject.setFileContent(fileContent);
```
最后,将FileObject对象传递到前端。可以使用Spring Boot的RestController注解来定义一个RESTful接口,将FileObject对象转换为JSON格式并返回给前端。例如:
```
@RestController
public class FileController {
@GetMapping("/file")
public FileObject getFile() {
File file = new File(filePath); // filePath为文件路径
String fileContent = new String(Files.readAllBytes(file.toPath()), StandardCharsets.UTF_8); // 读取文件内容
FileObject fileObject = new FileObject();
fileObject.setFileName(file.getName());
fileObject.setFilePath(filePath);
fileObject.setFileContent(fileContent);
return fileObject;
}
}
```
前端可以通过发送HTTP请求来调用这个接口,并获取FileObject对象。可以使用JavaScript的fetch API来发送GET请求并处理响应。例如:
```
fetch('/file')
.then(response => response.json())
.then(fileObject => {
console.log(fileObject);
// 在页面中显示文件内容等信息
});
```
阅读全文
相关推荐
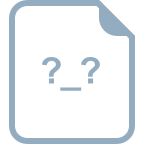
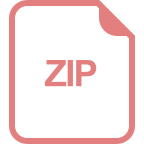
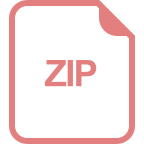
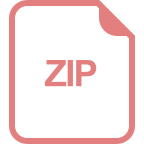
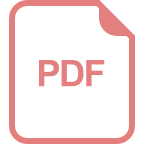
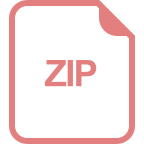
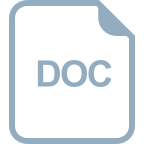
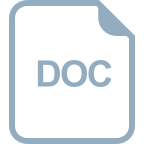
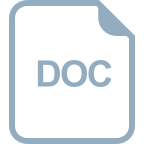
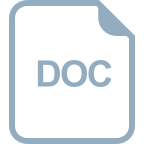
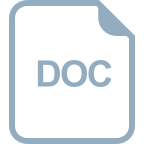
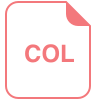
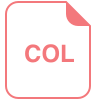

