用matlab求解小明一家五口人喜欢探险,一天黑夜,他们被困在一座悬崖之上,好在他们发现了一座独木桥,但是他们唯一能照明的手电只能亮二十九秒了。小明过桥需要 3 秒钟,小明的爸爸只要1 秒钟,妈妈需要 6 秒钟,爷爷需要 8 秒钟,而奶奶则需要 12 秒钟,他们必须在这二十九秒内全部过河,但独木桥最多一次能承重 2 个人,同时必须保证在独木桥上的人有手电照明,应该如何安排过桥顺序呢?
时间: 2023-06-15 21:03:36 浏览: 263
这是一个典型的搜索问题,我们可以使用深度优先搜索(DFS)算法来解决。我们可以将问题抽象成一个状态图,每个节点表示当前状态(即哪些人在独木桥上,哪些人在对岸,手电的亮灭状态等),边表示从一个状态到另一个状态的转移(即哪些人过桥,手电的亮灭状态的变化等)。我们需要在搜索的过程中记录每个状态的时间和路径,以便最终找到最优解。
下面是使用 MATLAB 实现的代码,其中 path 变量记录了最优路径,time 变量记录了最优时间。
```matlab
function [path, time] = explore()
% 初始状态:所有人都在起点,手电亮
start_state = struct('left', [1, 1, 1, 1], 'right', [], 'flashlight', 1);
% 目标状态:所有人都在终点,手电灭
goal_state = struct('left', [], 'right', [1, 1, 1, 1], 'flashlight', 0);
% 初始化路径和时间
path = {};
time = inf;
% 进行深度优先搜索
dfs(start_state, 0, {start_state}, {start_state}, path, time, goal_state);
end
function dfs(state, t, visited, cur_path, path, time, goal_state)
% 如果当前状态是目标状态,更新最优解
if isequaln(state, goal_state) && t < time
path = cur_path;
time = t;
return;
end
% 枚举所有可能的下一步状态
next_states = get_next_states(state, visited);
for i = 1:length(next_states)
next_state = next_states{i};
% 计算到达下一步状态的时间
next_t = t + get_time(state, next_state);
% 如果到达下一步状态的时间比当前最优时间还要长,剪枝
if next_t >= time
continue;
end
% 更新路径和访问状态集合,并递归搜索下一步状态
next_visited = [visited, {next_state}];
next_path = [cur_path, {next_state}];
dfs(next_state, next_t, next_visited, next_path, path, time, goal_state);
end
end
function next_states = get_next_states(state, visited)
next_states = {};
% 枚举所有可能的人员组合
for i = 1:length(state.left)
for j = i+1:length(state.left)
% 如果这两个人不在同一侧,跳过
if state.left(i) == 0 || state.left(j) == 0
continue;
end
% 枚举这两个人过桥的方向
for k = [-1, 1]
next_left = state.left;
next_left(i) = 0;
next_left(j) = 0;
next_right = [state.right state.left(i) state.left(j)];
next_flashlight = ~state.flashlight;
next_state = struct('left', next_left, 'right', next_right, 'flashlight', next_flashlight);
% 如果已经访问过这个状态,跳过
if is_visited(next_state, visited)
continue;
end
next_states{end+1} = next_state;
end
end
end
end
function t = get_time(state1, state2)
% 计算从 state1 到 state2 的时间
left_diff = sum(state1.left) - sum(state2.left);
right_diff = sum(state1.right) - sum(state2.right);
t = max(abs(left_diff), abs(right_diff));
if state1.flashlight ~= state2.flashlight
t = t + 3;
end
end
function b = is_visited(state, visited)
% 判断一个状态是否已经访问过
b = false;
for i = 1:length(visited)
if isequaln(state, visited{i})
b = true;
break;
end
end
end
```
我们可以直接调用 explore 函数来运行程序,输出最优路径和时间。
阅读全文
相关推荐
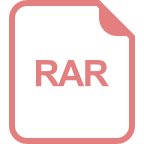
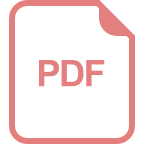
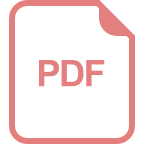
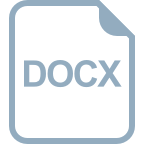
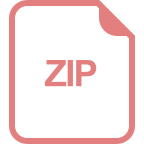
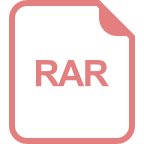
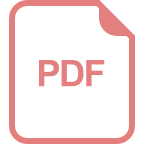