给定一个s字符串和一个n字符串,在s字符串中找出n字符串出现的第一个位置(从0开始)。如果不存在,则返回 -1 用c++
时间: 2024-02-10 08:10:44 浏览: 29
好的,以下是基于C++的代码实现,采用的是暴力匹配算法:
```
#include <iostream>
#include <string>
using namespace std;
int findFirstAppearance(string s, string n) {
int slen = s.length();
int nlen = n.length();
if (slen < nlen) {
return -1;
}
for (int i = 0; i <= slen - nlen; i++) {
bool flag = true;
for (int j = 0; j < nlen; j++) {
if (s[i + j] != n[j]) {
flag = false;
break;
}
}
if (flag) {
return i;
}
}
return -1;
}
int main() {
string s = "hello, world!";
string n = "world";
int pos = findFirstAppearance(s, n);
if (pos == -1) {
cout << "Not found" << endl;
} else {
cout << "Found at position " << pos << endl;
}
return 0;
}
```
这个方法的时间复杂度为 O(m*n),其中m为s字符串的长度,n为n字符串的长度。如果需要更高效的实现,可以采用KMP、BM、Sunday等算法来优化。
相关推荐
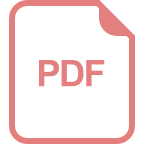






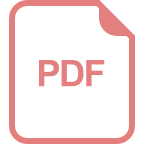
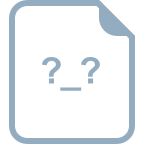
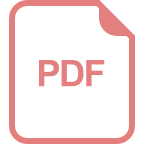
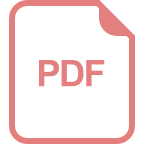
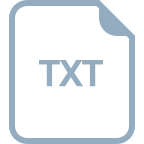