流水灯实验stm32f103
时间: 2023-07-29 09:05:57 浏览: 65
以下是使用 STM32F103 单片机实现流水灯实验的代码,使用的是 Keil MDK-ARM 编译器:
```
#include "stm32f10x.h"
// 定义 LED 灯的 GPIO 引脚号
#define LED1_PIN GPIO_Pin_12
#define LED2_PIN GPIO_Pin_13
#define LED3_PIN GPIO_Pin_14
#define LED4_PIN GPIO_Pin_15
// 定义 LED 灯的 GPIO 端口号
#define LED1_PORT GPIOB
#define LED2_PORT GPIOB
#define LED3_PORT GPIOB
#define LED4_PORT GPIOB
// 定义定时器 2 的频率和分频系数
#define TIM2_FREQ 10000
#define TIM2_PSC 71
// 定义定时器 2 中断处理函数
void TIM2_IRQHandler()
{
static uint8_t cnt = 0;
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET) {
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
cnt++;
if (cnt == 1) {
GPIO_SetBits(LED1_PORT, LED1_PIN);
GPIO_ResetBits(LED2_PORT, LED2_PIN);
GPIO_ResetBits(LED3_PORT, LED3_PIN);
GPIO_ResetBits(LED4_PORT, LED4_PIN);
} else if (cnt == 2) {
GPIO_ResetBits(LED1_PORT, LED1_PIN);
GPIO_SetBits(LED2_PORT, LED2_PIN);
GPIO_ResetBits(LED3_PORT, LED3_PIN);
GPIO_ResetBits(LED4_PORT, LED4_PIN);
} else if (cnt == 3) {
GPIO_ResetBits(LED1_PORT, LED1_PIN);
GPIO_ResetBits(LED2_PORT, LED2_PIN);
GPIO_SetBits(LED3_PORT, LED3_PIN);
GPIO_ResetBits(LED4_PORT, LED4_PIN);
} else if (cnt == 4) {
GPIO_ResetBits(LED1_PORT, LED1_PIN);
GPIO_ResetBits(LED2_PORT, LED2_PIN);
GPIO_ResetBits(LED3_PORT, LED3_PIN);
GPIO_SetBits(LED4_PORT, LED4_PIN);
cnt = 0;
}
}
}
// 初始化 GPIO
void GPIO_Init()
{
GPIO_InitTypeDef GPIO_InitStruct;
// 使能 GPIOB 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置 LED1 引脚为推挽输出模式
GPIO_InitStruct.GPIO_Pin = LED1_PIN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED1_PORT, &GPIO_InitStruct);
// 配置 LED2 引脚为推挽输出模式
GPIO_InitStruct.GPIO_Pin = LED2_PIN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED2_PORT, &GPIO_InitStruct);
// 配置 LED3 引脚为推挽输出模式
GPIO_InitStruct.GPIO_Pin = LED3_PIN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED3_PORT, &GPIO_InitStruct);
// 配置 LED4 引脚为推挽输出模式
GPIO_InitStruct.GPIO_Pin = LED4_PIN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED4_PORT, &GPIO_InitStruct);
}
// 初始化定时器 2
void TIM2_Init()
{
TIM_TimeBaseInitTypeDef TIM_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
// 使能定时器 2 时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
// 配置定时器 2
TIM_InitStruct.TIM_Period = (SystemCoreClock / (TIM2_FREQ * TIM2_PSC)) - 1;
TIM_InitStruct.TIM_Prescaler = TIM2_PSC - 1;
TIM_InitStruct.TIM_ClockDivision = 0;
TIM_InitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_InitStruct);
// 配置定时器 2 中断
NVIC_InitStruct.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
// 启动定时器 2
TIM_Cmd(TIM2, ENABLE);
// 启动定时器 2 中断
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
}
// 主函数
int main()
{
// 初始化 GPIO 和定时器 2
GPIO_Init();
TIM2_Init();
while (1) {
// 主程序空转
}
}
```
以上代码实现了四个 LED 灯的流水灯效果,使用定时器中断控制灯的亮灭时间,可以在 STM32F103 单片机开发板上测试。
相关推荐
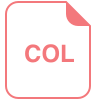
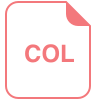












