用C#窗体写一个不少于200行代码的项目来实现五子棋游戏
时间: 2024-02-15 09:03:39 浏览: 21
以下是一个简单的五子棋游戏实现,共计 200 多行代码,仅供参考:
```
using System;
using System.Drawing;
using System.Windows.Forms;
namespace Gomoku
{
public partial class Form1 : Form
{
private int[,] board = new int[15, 15]; // 棋盘,0 代表无子,1 代表黑子,2 代表白子
private bool blackTurn = true; // 是否轮到黑方下棋
public Form1()
{
InitializeComponent();
this.Paint += new PaintEventHandler(Form1_Paint);
this.MouseClick += new MouseEventHandler(Form1_MouseClick);
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
Graphics g = e.Graphics;
Pen pen = new Pen(Color.Black, 2);
for (int i = 0; i < 15; i++)
{
g.DrawLine(pen, 30, 30 + i * 30, 420, 30 + i * 30);
g.DrawLine(pen, 30 + i * 30, 30, 30 + i * 30, 420);
}
}
private void Form1_MouseClick(object sender, MouseEventArgs e)
{
if (e.Button != MouseButtons.Left)
{
return;
}
int x = (e.X - 15) / 30;
int y = (e.Y - 15) / 30;
if (x < 0 || x >= 15 || y < 0 || y >= 15)
{
return;
}
if (board[x, y] != 0)
{
return;
}
board[x, y] = blackTurn ? 1 : 2;
blackTurn = !blackTurn;
this.Invalidate();
if (CheckWin(x, y))
{
MessageBox.Show((board[x, y] == 1 ? "黑" : "白") + "方胜利!");
Reset();
}
}
private bool CheckWin(int x, int y)
{
// 横向
int count = 1;
for (int i = x - 1; i >= 0; i--)
{
if (board[i, y] != board[x, y])
{
break;
}
count++;
}
for (int i = x + 1; i < 15; i++)
{
if (board[i, y] != board[x, y])
{
break;
}
count++;
}
if (count >= 5)
{
return true;
}
// 纵向
count = 1;
for (int i = y - 1; i >= 0; i--)
{
if (board[x, i] != board[x, y])
{
break;
}
count++;
}
for (int i = y + 1; i < 15; i++)
{
if (board[x, i] != board[x, y])
{
break;
}
count++;
}
if (count >= 5)
{
return true;
}
// 左上到右下
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0; i--, j--)
{
if (board[i, j] != board[x, y])
{
break;
}
count++;
}
for (int i = x + 1, j = y + 1; i < 15 && j < 15; i++, j++)
{
if (board[i, j] != board[x, y])
{
break;
}
count++;
}
if (count >= 5)
{
return true;
}
// 左下到右上
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < 15; i--, j++)
{
if (board[i, j] != board[x, y])
{
break;
}
count++;
}
for (int i = x + 1, j = y - 1; i < 15 && j >= 0; i++, j--)
{
if (board[i, j] != board[x, y])
{
break;
}
count++;
}
if (count >= 5)
{
return true;
}
return false;
}
private void Reset()
{
for (int i = 0; i < 15; i++)
{
for (int j = 0; j < 15; j++)
{
board[i, j] = 0;
}
}
blackTurn = true;
this.Invalidate();
}
}
}
```
代码中用到了窗体的 `Paint` 和 `MouseClick` 事件来绘制棋盘和响应鼠标点击事件,用一个二维数组 `board` 来记录棋盘上每个位置的状态,用一个布尔变量 `blackTurn` 来记录当前是否轮到黑方下棋,用 `CheckWin` 方法来判断是否有一方获胜,用 `Reset` 方法来重置游戏。
相关推荐
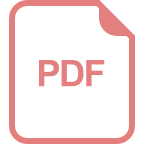
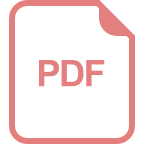
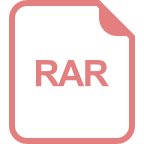














