Connection con = null; Statement st = null; ResultSet rs = null;
时间: 2024-03-22 07:39:44 浏览: 61
这是Java中用于连接数据库并执行SQL语句的基本代码模板。它包括三个变量:
1. Connection对象:用于与数据库建立连接。
2. Statement对象:用于执行SQL语句。
3. ResultSet对象:用于存储从数据库检索的数据。
通常情况下,我们会使用try-catch-finally语句块来处理与数据库的交互。在try块中,我们建立连接、执行SQL语句并获取结果集。在catch块中,我们处理任何可能发生的异常。在finally块中,我们关闭ResultSet、Statement和Connection对象以释放资源。
示例代码:
```
try {
// 建立连接
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// 执行SQL语句
st = con.createStatement();
rs = st.executeQuery("SELECT * FROM mytable");
// 处理结果集
while (rs.next()) {
// 从结果集中获取数据
int id = rs.getInt("id");
String name = rs.getString("name");
float price = rs.getFloat("price");
System.out.println(id + "\t" + name + "\t" + price);
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
// 关闭资源
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (st != null) {
try {
st.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (con != null) {
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们使用MySQL数据库,并使用JDBC API来建立连接、执行SQL语句和处理结果集。在finally块中,我们使用if-else语句来检查每个对象是否为null,并在确保不为空的情况下关闭它们。
阅读全文
相关推荐















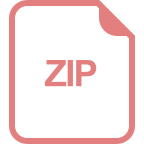