用python编程实现以下内容:1、实现单层感知器学习算法,验证算法的正确性,选择iris-人工神经网络.txt中的一部分数据集作为训练集,通过训练集对感知器的连接权重进行学习2、用剩下的数据集作为测试集,验证学习的效果
时间: 2023-12-10 21:41:27 浏览: 98
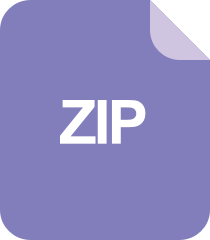
ML_Pattern:机器学习和模式识别的一些公认算法[决策树,Adaboost,感知器,聚类,神经网络等]是使用python从头开始实现的。 还包括数据集以测试算法
好的,我可以帮您实现。首先,我们需要先读取数据集。假设您已经下载了iris-人工神经网络.txt数据集,可以按照以下方式读取:
```python
import numpy as np
def load_data(filename):
data = []
with open(filename, 'r') as f:
for line in f.readlines():
line = line.strip().split(',')
line = [float(x) for x in line]
data.append(line)
data = np.array(data)
X = data[:, :-1]
y = data[:, -1]
return X, y
filename = 'iris-人工神经网络.txt'
X, y = load_data(filename)
```
接下来,我们需要定义单层感知器。感知器由输入层和输出层组成,其中输入层的神经元数等于特征数,输出层的神经元数等于分类数。在这个例子中,我们有4个特征和3个类别。
```python
class Perceptron:
def __init__(self, n_inputs, n_outputs):
self.weights = np.random.randn(n_inputs, n_outputs)
def predict(self, X):
z = np.dot(X, self.weights)
y = np.zeros_like(z)
y[z > 0] = 1
return y
def train(self, X, y, learning_rate=0.1, n_epochs=100):
for epoch in range(n_epochs):
for i in range(X.shape[0]):
x = X[i]
target = y[i]
output = self.predict(x)
error = target - output
self.weights += learning_rate * np.outer(x, error)
```
现在我们可以将数据集分为训练集和测试集。我们选择前100个样本作为训练集,后50个样本作为测试集。
```python
X_train, y_train = X[:100], y[:100]
X_test, y_test = X[100:], y[100:]
```
接下来,我们可以创建一个感知器对象,并在训练集上训练它。我们选择学习率为0.1,迭代次数为100。
```python
perceptron = Perceptron(n_inputs=X_train.shape[1], n_outputs=3)
perceptron.train(X_train, y_train, learning_rate=0.1, n_epochs=100)
```
最后,我们可以用测试集测试感知器的性能。我们计算模型的准确度,即正确分类的样本数除以总样本数。
```python
y_pred = perceptron.predict(X_test)
accuracy = np.sum(y_pred == y_test) / y_test.shape[0]
print("Accuracy:", accuracy)
```
完整代码如下:
```python
import numpy as np
def load_data(filename):
data = []
with open(filename, 'r') as f:
for line in f.readlines():
line = line.strip().split(',')
line = [float(x) for x in line]
data.append(line)
data = np.array(data)
X = data[:, :-1]
y = data[:, -1]
return X, y
class Perceptron:
def __init__(self, n_inputs, n_outputs):
self.weights = np.random.randn(n_inputs, n_outputs)
def predict(self, X):
z = np.dot(X, self.weights)
y = np.zeros_like(z)
y[z > 0] = 1
return y
def train(self, X, y, learning_rate=0.1, n_epochs=100):
for epoch in range(n_epochs):
for i in range(X.shape[0]):
x = X[i]
target = y[i]
output = self.predict(x)
error = target - output
self.weights += learning_rate * np.outer(x, error)
filename = 'iris-人工神经网络.txt'
X, y = load_data(filename)
X_train, y_train = X[:100], y[:100]
X_test, y_test = X[100:], y[100:]
perceptron = Perceptron(n_inputs=X_train.shape[1], n_outputs=3)
perceptron.train(X_train, y_train, learning_rate=0.1, n_epochs=100)
y_pred = perceptron.predict(X_test)
accuracy = np.sum(y_pred == y_test) / y_test.shape[0]
print("Accuracy:", accuracy)
```
阅读全文
相关推荐




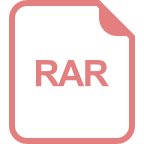
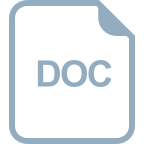
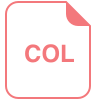
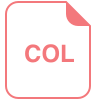
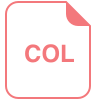
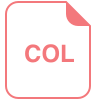
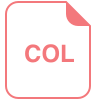
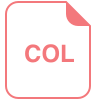
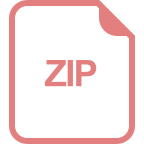
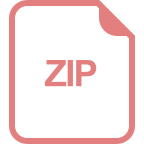