点云切片求交法代码
时间: 2023-07-23 08:13:16 浏览: 303
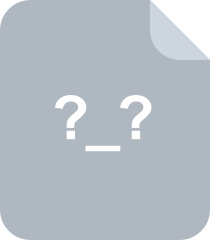
点云切片C++代码 求取点云体积的上一步

以下是一个简单的点云切片求交法的 Python 代码示例:
```python
import numpy as np
def plane_normal(point1, point2, point3):
# 计算平面法向量
v1 = point2 - point1
v2 = point3 - point1
normal = np.cross(v1, v2)
return normal / np.linalg.norm(normal)
def point_plane_intersection(point, normal, plane_point):
# 计算点与平面的交点
t = np.dot(normal, plane_point - point) / np.dot(normal, normal)
intersection = point + t * normal
return intersection
def polygon_plane_intersection(polygon, normal, plane_point):
# 计算多边形与平面的交点
intersections = []
for i in range(len(polygon)):
j = (i + 1) % len(polygon)
edge_start = polygon[i]
edge_end = polygon[j]
edge_direction = edge_end - edge_start
t = np.dot(normal, plane_point - edge_start) / np.dot(normal, edge_direction)
if t >= 0 and t <= 1:
intersection = edge_start + t * edge_direction
intersections.append(intersection)
return intersections
def slice_point_cloud(point_cloud, plane_point, plane_normal):
# 将点云投影到切片平面上,并计算点云与平面的交点
projected_points = []
intersections = []
for point in point_cloud:
projected_point = point_plane_intersection(point, plane_normal, plane_point)
projected_points.append(projected_point)
intersection = point_plane_intersection(point, plane_normal, plane_point)
intersections.append(intersection)
# 将投影点集按照顺序连成一条封闭的多边形
polygon = np.array(projected_points)
# 计算多边形与平面的交点
intersections = polygon_plane_intersection(polygon, plane_normal, plane_point)
return intersections
```
该代码实现了一个简单的点云切片求交法,包括计算平面法向量、点与平面的交点、多边形与平面的交点,以及将点云投影到切片平面上并计算点云与平面的交点等功能。使用该代码可以计算点云与任意平面的相交情况,具体使用方法可以参考以下示例:
```python
# 创建一个随机点云
point_cloud = np.random.rand(100, 3)
# 定义一个平面
plane_point = np.array([0, 0, 0])
plane_normal = np.array([1, 0, 0])
# 计算点云与平面的交点
intersections = slice_point_cloud(point_cloud, plane_point, plane_normal)
```
阅读全文
相关推荐
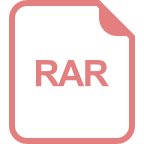

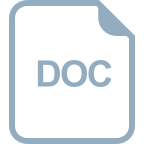
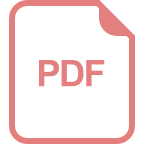
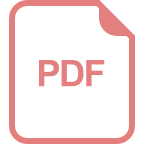
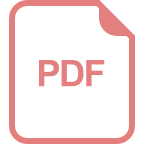
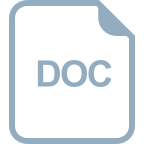
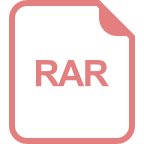
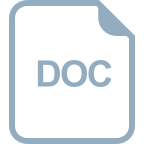
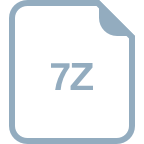
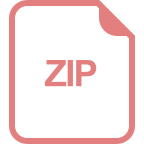
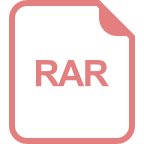
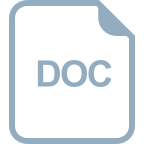

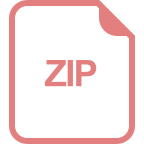