pyopengl如何创建着色器绘制多个点
时间: 2024-06-09 07:05:14 浏览: 161
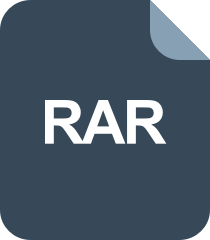
用Opengl实现多边形的绘制

要在 PyOpenGL 中创建着色器并绘制多个点,可以按照以下步骤进行:
1. 导入必要的库
```python
from OpenGL.GL import *
from OpenGL.GL.shaders import compileProgram, compileShader
import numpy as np
```
2. 编写顶点着色器和片元着色器
```python
vertex_shader = """
#version 330
in vec4 position;
void main()
{
gl_Position = position;
}
"""
fragment_shader = """
#version 330
void main()
{
gl_FragColor = vec4(1.0, 1.0, 1.0, 1.0);
}
"""
```
3. 编译着色器程序
```python
shader = compileProgram(compileShader(vertex_shader, GL_VERTEX_SHADER), compileShader(fragment_shader, GL_FRAGMENT_SHADER))
```
4. 创建顶点数据并绑定到缓冲区对象
```python
vertices = np.array([0.0, 0.5, 0.0, 1.0,
-0.5, -0.5, 0.0, 1.0,
0.5, -0.5, 0.0, 1.0], dtype=np.float32)
vbo = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, vbo)
glBufferData(GL_ARRAY_BUFFER, vertices.nbytes, vertices, GL_STATIC_DRAW)
```
5. 启用顶点属性并指定其数据格式
```python
position = glGetAttribLocation(shader, "position")
glEnableVertexAttribArray(position)
glVertexAttribPointer(position, 4, GL_FLOAT, GL_FALSE, 0, None)
```
6. 使用着色器程序并绘制多个点
```python
glUseProgram(shader)
glDrawArrays(GL_POINTS, 0, 3)
```
完整代码如下:
```python
from OpenGL.GL import *
from OpenGL.GL.shaders import compileProgram, compileShader
import numpy as np
import glfw
vertex_shader = """
#version 330
in vec4 position;
void main()
{
gl_Position = position;
}
"""
fragment_shader = """
#version 330
void main()
{
gl_FragColor = vec4(1.0, 1.0, 1.0, 1.0);
}
"""
def main():
if not glfw.init():
return
window = glfw.create_window(640, 480, "PyOpenGL Demo", None, None)
if not window:
glfw.terminate()
return
glfw.make_context_current(window)
shader = compileProgram(compileShader(vertex_shader, GL_VERTEX_SHADER), compileShader(fragment_shader, GL_FRAGMENT_SHADER))
vertices = np.array([0.0, 0.5, 0.0, 1.0,
-0.5, -0.5, 0.0, 1.0,
0.5, -0.5, 0.0, 1.0], dtype=np.float32)
vbo = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, vbo)
glBufferData(GL_ARRAY_BUFFER, vertices.nbytes, vertices, GL_STATIC_DRAW)
position = glGetAttribLocation(shader, "position")
glEnableVertexAttribArray(position)
glVertexAttribPointer(position, 4, GL_FLOAT, GL_FALSE, 0, None)
while not glfw.window_should_close(window):
glfw.poll_events()
glClear(GL_COLOR_BUFFER_BIT)
glUseProgram(shader)
glDrawArrays(GL_POINTS, 0, 3)
glfw.swap_buffers(window)
glfw.terminate()
if __name__ == '__main__':
main()
```
这个程序将创建一个包含三个点的三角形,并以白色绘制它们。你可以使用 `glDrawArrays` 函数中的第二个参数来指定要绘制的点的数量。
阅读全文
相关推荐
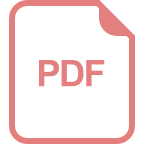
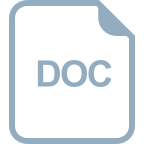
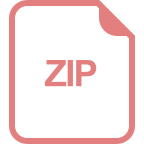
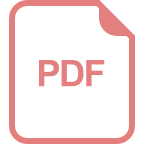
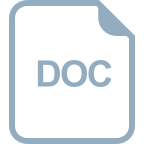
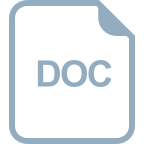
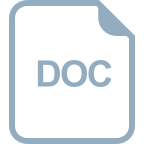
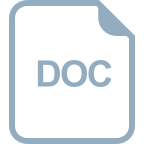
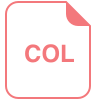
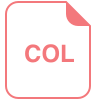




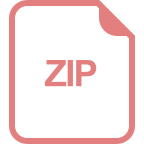