用python写出RF对csv特征文件进行筛选
时间: 2023-08-31 11:18:32 浏览: 65
### 回答1:
我可以为你提供一些参考,你可以使用Python的Pandas包来对CSV文件进行特征筛选,例如:import pandas as pd df = pd.read_csv('my_data.csv') # Create a list of features to keep features_to_keep = ['feature1', 'feature2', 'feature3'] # Get names of features in the csv file all_features = df.columns.values # Keep only the desired features df = df[features_to_keep]
### 回答2:
使用Python编写RF(随机森林)算法可以对CSV特征文件进行筛选。下面是一个简单的步骤:
1. 导入必要的库和模块:
```python
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.feature_selection import SelectFromModel
```
2. 读取CSV文件并将其转换为DataFrame:
```python
data = pd.read_csv('feature_file.csv')
```
3. 将特征和目标(如果有的话)分别提取出来:
```python
X = data.iloc[:, :-1] # 特征
y = data.iloc[:, -1] # 目标
```
4. 初始化随机森林分类器:
```python
clf = RandomForestClassifier(n_estimators=100)
```
5. 使用随机森林分类器拟合数据:
```python
clf.fit(X, y)
```
6. 使用SelectFromModel方法基于特征的重要性进行特征选择:
```python
sfm = SelectFromModel(clf, threshold=0.2) # 根据重要性阈值选择特征
sfm.fit(X, y)
selected_features = X.columns[sfm.get_support()] # 获取选择的特征列名
```
7. 打印被选中的特征:
```python
print(selected_features)
```
以上是使用Python编写RF对CSV特征文件进行筛选的步骤。你可以根据自己的需求和数据进行相应的调整和修改。
### 回答3:
RF(Random Forest)是一种常用的机器学习算法,可用于特征选择。使用Python编写代码对csv特征文件进行筛选的步骤如下:
1. 导入所需的库和模块:
```python
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
```
2. 读取CSV文件并获取特征矩阵X和目标变量y:
```python
data = pd.read_csv('filename.csv')
X = data.iloc[:, :-1] # 特征矩阵
y = data.iloc[:, -1] # 目标变量
```
3. 创建Random Forest分类器对象,并拟合数据:
```python
rf = RandomForestClassifier()
rf.fit(X, y)
```
4. 获取特征的重要性排序:
```python
importances = rf.feature_importances_
indices = np.argsort(importances)[::-1]
```
5. 可选:可视化特征重要性排序结果:
```python
import matplotlib.pyplot as plt
plt.figure()
plt.title("Feature Importance")
plt.bar(range(X.shape[1]), importances[indices])
plt.xticks(range(X.shape[1]), indices)
plt.show()
```
6. 根据需要保留重要性较高的特征,以进行筛选。
请替换'filename.csv'为实际的文件名,并根据数据的具体情况进行相应的修改。这样,你就可以使用Python对CSV特征文件进行筛选。
相关推荐
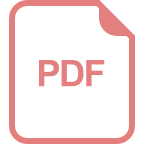














