好的,那么我们可以对这段代码进行一些修改和完善,以提高其安全性和可读性。具体来说,可以考虑以下几个方面: 1. 使用安全的输入方式 gets函数是一个不安全的函数,可能会导致缓冲区溢出等问题。建议使用更加安全的输入方式,例如使用std::cin替代gets。同时,需要注意输入的长度不能超过缓冲区的大小。 2. 完善函数的实现 这段代码中的一些函数并没有完整的实现,例如Calculate函数和CalculateOut函数。为了保证计算器的正确性和稳定性,需要对这些函数进行完善。 3. 增加注释和说明 为了方便其他人阅读和理解这段代码,建议增加适当的注释和说明。这样可以使代码更加易读和易懂,也有助于其他人学习和使用这段代码。 4. 改进变量命名和代码结构 为了提高代码的可读性和可维护性,建议改进变量命名和代码结构。变量命名应该具有描述性,能够清晰地表达其用途。同时,代码结构应该清晰明了,遵循良好的编程规范和习惯。 综上所述,如果您确实需要使用这段代码,请在使用之前进行必要的修改和完善,以提高其安全性和可读性。
时间: 2024-04-06 21:32:42 浏览: 46
非常好的建议!这些改进可以使代码更加可靠和易于维护。以下是对每个方面的更详细的说明:
1. 使用安全的输入方式
使用std::cin替代gets函数可以避免缓冲区溢出等问题。我们可以使用std::cin.getline()函数来读取输入,使其更加安全可靠。例如,可以使用以下代码:
```c++
char input[256];
std::cin.getline(input, 256);
```
2. 完善函数的实现
在Calculate函数和CalculateOut函数中,我们需要确保计算器的正确性和稳定性。可以通过添加边界检查和错误处理来实现。例如,可以在Calculate函数中添加以下代码:
```c++
double Calculate(char op, double num1, double num2)
{
double result = 0.0;
switch(op)
{
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if(num2 == 0)
{
std::cerr << "Error: Division by zero" << std::endl;
exit(EXIT_FAILURE);
}
result = num1 / num2;
break;
default:
std::cerr << "Error: Invalid operator" << std::endl;
exit(EXIT_FAILURE);
}
return result;
}
```
3. 增加注释和说明
添加注释和说明可以帮助其他人更好地理解代码。例如,我们可以在每个函数和变量声明的上方添加注释来描述其用途和功能。同时,我们也可以在代码中添加注释来解释一些复杂的逻辑和算法。以下是一个示例:
```c++
// This function calculates the result of an operation between two numbers
// op: the operator (+, -, *, /)
// num1: the first number
// num2: the second number
// returns: the result of the operation
double Calculate(char op, double num1, double num2)
{
// ...
}
// This function converts a string to a double
// str: the string to be converted
// returns: the converted double value
double ConvertToDouble(const std::string& str)
{
// ...
}
int main()
{
// ...
}
```
4. 改进变量命名和代码结构
良好的变量命名和代码结构可以使代码更加易读和易于维护。变量名应该具有描述性,能够清晰地表达其用途。代码结构应该清晰明了,遵循良好的编程规范和习惯。以下是一些示例:
```c++
double Calculate(char op, double num1, double num2)
{
double result = 0.0;
switch(op)
{
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if(num2 == 0)
{
std::cerr << "Error: Division by zero" << std::endl;
exit(EXIT_FAILURE);
}
result = num1 / num2;
break;
default:
std::cerr << "Error: Invalid operator" << std::endl;
exit(EXIT_FAILURE);
}
return result;
}
double ConvertToDouble(const std::string& str)
{
double result = 0.0;
try
{
result = std::stod(str);
}
catch(const std::exception& e)
{
std::cerr << "Error: Invalid input" << std::endl;
exit(EXIT_FAILURE);
}
return result;
}
int main()
{
std::cout << "Enter an expression: " << std::endl;
char input[256];
std::cin.getline(input, 256);
std::string str(input);
std::istringstream iss(str);
double num1 = 0.0;
double num2 = 0.0;
char op = '\0';
iss >> num1 >> op >> num2;
double result = Calculate(op, num1, num2);
std::cout << "Result: " << result << std::endl;
return 0;
}
```
综上所述,我们可以通过上述的改进来提高代码的可靠性和易于维护性。这些改进可以帮助我们编写更好的代码,避免一些潜在的安全问题,同时也可以使代码更加易于理解和修改。
阅读全文
相关推荐
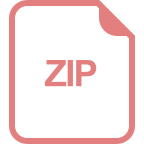
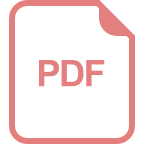
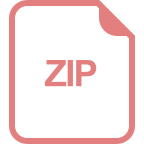
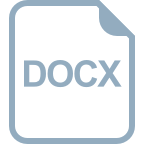
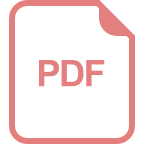
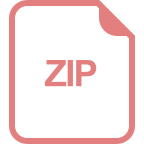
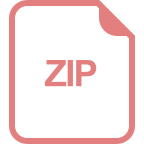
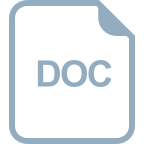
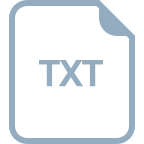
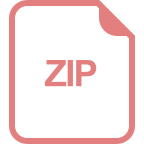
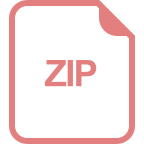
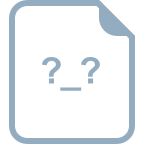
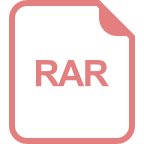
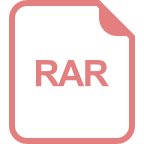
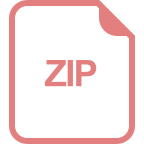
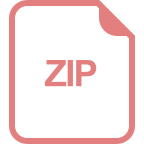
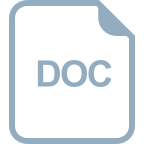