请用C语言实现strstr函数,至少使用两种方法
时间: 2024-05-02 12:21:18 浏览: 22
方法1:暴力匹配
```c
char* my_strstr(char* haystack, char* needle) {
if (!*needle) return haystack;
char* p1 = haystack;
while (*p1) {
char* p1_begin = p1, *p2 = needle;
while (*p1 && *p2 && *p1 == *p2) {
p1++;
p2++;
}
if (!*p2) return p1_begin;
p1 = p1_begin + 1;
}
return NULL;
}
```
方法2:KMP算法
```c
void compute_prefix(char* pattern, int* prefix) {
int i = 1, j = 0;
prefix[0] = 0;
while (pattern[i]) {
if (pattern[i] == pattern[j]) {
j++;
prefix[i] = j;
i++;
} else if (j > 0) {
j = prefix[j - 1];
} else {
prefix[i] = 0;
i++;
}
}
}
char* my_strstr(char* haystack, char* needle) {
if (!*needle) return haystack;
int n = strlen(haystack), m = strlen(needle);
int prefix[m];
compute_prefix(needle, prefix);
int i = 0, j = 0;
while (i < n) {
if (haystack[i] == needle[j]) {
i++;
j++;
} else if (j > 0) {
j = prefix[j - 1];
} else {
i++;
}
if (j == m) return haystack + i - m;
}
return NULL;
}
```
相关推荐
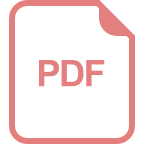












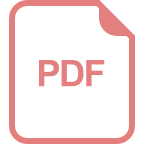
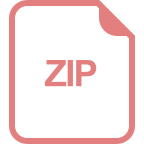
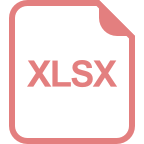