Python Ftplib遍历目录指定文件
时间: 2023-11-30 12:02:16 浏览: 92
可以使用 `ftplib` 模块的 `NLST()` 方法获取指定目录下的文件列表,然后遍历列表,找到指定文件即可。
以下是一个简单的示例代码:
```python
import ftplib
# 连接 FTP 服务器
ftp = ftplib.FTP("ftp.example.com")
ftp.login("username", "password")
# 进入指定目录
ftp.cwd("/path/to/directory")
# 获取指定目录下的文件列表
file_list = ftp.nlst()
# 遍历文件列表,找到指定文件
for file_name in file_list:
if file_name.endswith(".txt"): # 指定文件名或者后缀
print("Found file:", file_name)
# 关闭 FTP 连接
ftp.quit()
```
说明:
- `ftp.nlst()` 返回指定目录下的文件列表。
- `str.endswith(suffix)` 方法判断字符串是否以指定的后缀结尾。
相关问题
在Python的ftplib库中,并没有直接提供删除目录子目录及文件的功能。但是,你可以通过先遍历目录内容,然后逐个删除文件和子目录来实现这一操作
在Python的`ftplib`库中,它本身并没有直接提供删除整个目录及其内容的功能。`FTP`对象提供的是一些基础的文件操作,如`listdir()`用于获取目录列表,`delete()`用于删除单个文件,`rmdir()`用于删除空目录。
如果你想要删除目录树(包括目录和文件),你需要自己编写一些代码来实现这个过程。通常的做法是先使用`listdir()`获取目录下的所有文件和子目录,然后对每个子项递归地调用删除方法。下面是一个简单的示例:
```python
import os
import ftplib
def delete_recursive(ftp, dir_path):
# 确保在正确的目录下
ftp.cwd(dir_path)
# 获取目录内容
files = ftp.nlst()
for file_or_subdir in files:
full_path = os.path.join(dir_path, file_or_subdir)
if os.path.isdir(full_path): # 如果是子目录
delete_recursive(ftp, full_path) # 递归删除子目录
else: # 如果是文件
try:
ftp.delete(file_or_subdir)
print(f'Deleted: {full_path}')
except ftplib.all_errors as e:
print(f'Error deleting {full_path}: {str(e)}')
# 连接到FTP服务器
ftp = ftplib.FTP('your_host', 'username', 'password')
ftp.login()
# 删除指定目录及其内容
delete_recursive(ftp, '/your/directory/path')
ftp.quit()
```
这段代码假设FTP服务器允许删除目录和文件。请注意,在实际应用中,你可能需要根据服务器的配置和限制进行相应的调整。
python使用ftplib将本地文件夹传送到指定目录
在Python中,你可以使用`ftplib`模块来创建FTP连接,并通过`os`模块来处理本地文件夹的内容。以下是基本步骤:
首先,你需要导入所需的库:
```python
import os
import ftplib
```
然后定义函数,该函数会连接到FTP服务器,上传文件夹内容:
```python
def upload_folder_to_ftp(local_dir, remote_dir, ftp_server, username, password):
# 创建FTP客户端对象
ftp = ftplib.FTP(ftp_server)
# 认证
ftp.login(username, password)
try:
# 进入远程目录
ftp.cwd(remote_dir)
# 遍历本地文件夹并上传文件
for filename in os.listdir(local_dir):
local_path = os.path.join(local_dir, filename)
with open(local_path, 'rb') as file:
remote_path = os.path.join(remote_dir, filename) # 构造远程路径
ftp.storbinary(f'STOR {remote_path}', file) # 二进制模式上传
print("文件夹上传完成")
except Exception as e:
print(f"上传过程中发生错误:{e}")
finally:
# 断开FTP连接
ftp.quit()
```
要在本地文件夹`local_dir`下所有子目录和文件上传到FTP服务器上指定目录`remote_dir`,你可以这样调用这个函数:
```python
upload_folder_to_ftp('/path/to/local/dir', '/remote/directory/', 'your_username', 'your_password')
```
阅读全文
相关推荐
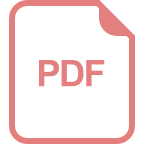
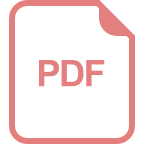
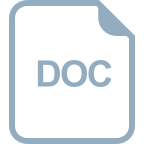
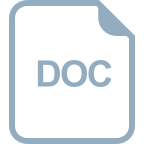
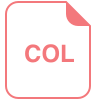
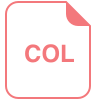
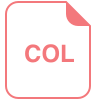
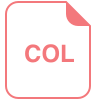
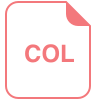
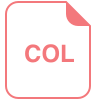
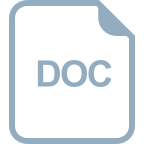
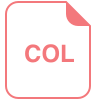
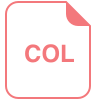
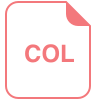
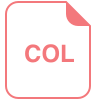
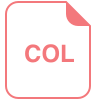