AJA在本地的服务器上创建一个mysql类型的数据库,叫register, 库中有一个名为user的数据表,包括以下字段:id(主键,自增), username, trueName, password, sex, age, favor, province, intro;创建一个html页面呈现用户注册表单,并对用户注册表单进行适当的美化,如各个表单元素对齐等;后台一个php页面,用于将注册数据的插入到数据库中。要求必须用jq ajax相关技术实现。
时间: 2024-02-29 18:52:15 浏览: 144
好的,让我来回答你的问题。
首先,关于创建数据库和数据表,可以使用以下的 SQL 语句:
```sql
CREATE DATABASE register;
USE register;
CREATE TABLE user (
id INT(11) PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
trueName VARCHAR(50) NOT NULL,
password VARCHAR(50) NOT NULL,
sex VARCHAR(10),
age INT(11),
favor VARCHAR(50),
province VARCHAR(50),
intro VARCHAR(255)
);
```
接下来是前端页面的设计,可以使用以下代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>用户注册表单</title>
<style>
form {
max-width: 500px;
margin: 0 auto;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"], input[type="password"], select {
width: 100%;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
margin-bottom: 10px;
}
input[type="submit"] {
background-color: #4CAF50;
color: white;
padding: 12px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
float: right;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<form id="register-form">
<label for="username">用户名:</label>
<input type="text" id="username" name="username" required>
<label for="trueName">真实姓名:</label>
<input type="text" id="trueName" name="trueName" required>
<label for="password">密码:</label>
<input type="password" id="password" name="password" required>
<label for="sex">性别:</label>
<select id="sex" name="sex">
<option value="">请选择</option>
<option value="男">男</option>
<option value="女">女</option>
</select>
<label for="age">年龄:</label>
<input type="text" id="age" name="age">
<label for="favor">爱好:</label>
<input type="text" id="favor" name="favor">
<label for="province">省份:</label>
<input type="text" id="province" name="province">
<label for="intro">个人介绍:</label>
<textarea id="intro" name="intro"></textarea>
<input type="submit" value="注册">
</form>
<script>
$(function() {
$('#register-form').submit(function(e) {
e.preventDefault();
$.ajax({
type: 'POST',
url: 'register.php',
data: $(this).serialize(),
success: function(data) {
alert('注册成功!');
},
error: function() {
alert('注册失败!');
}
});
});
});
</script>
</body>
</html>
```
最后是后端的 PHP 页面,可以使用以下代码:
```php
<?php
$servername = "localhost";
$username = "root";
$password = "password";
$dbname = "register";
// 创建连接
$conn = new mysqli($servername, $username, $password, $dbname);
// 检查连接
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
// 获取表单提交的数据
$username = $_POST['username'];
$trueName = $_POST['trueName'];
$password = $_POST['password'];
$sex = $_POST['sex'];
$age = $_POST['age'];
$favor = $_POST['favor'];
$province = $_POST['province'];
$intro = $_POST['intro'];
// 插入数据
$sql = "INSERT INTO user (username, trueName, password, sex, age, favor, province, intro)
VALUES ('$username', '$trueName', '$password', '$sex', '$age', '$favor', '$province', '$intro')";
if ($conn->query($sql) === TRUE) {
echo "插入成功";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
?>
```
希望以上代码能够帮到你。
阅读全文
相关推荐
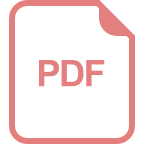
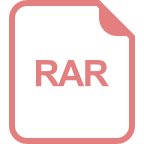
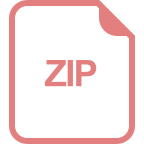
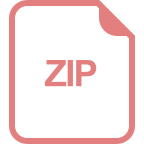
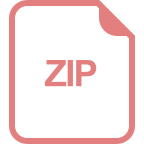
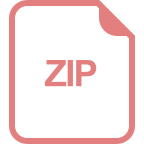
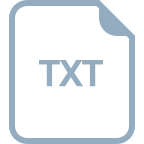
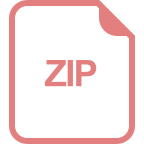
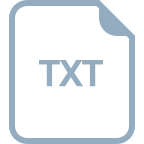
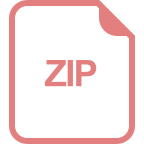
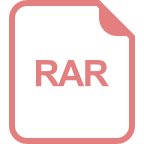
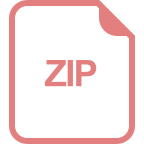
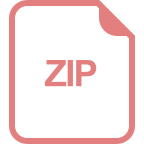
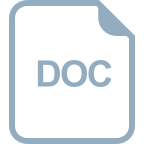
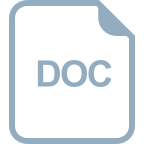