CameraPreview 添加时间水印
时间: 2023-07-21 17:30:26 浏览: 54
要在 CameraPreview 中添加时间水印,可以使用 SurfaceView 来预览摄像头,并在 SurfaceView 上绘制时间水印。以下是一个简单的示例:
1. 在布局文件中添加一个 SurfaceView 元素,用于显示摄像头预览和录制的视频:
```xml
<SurfaceView
android:id="@+id/surfaceView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2. 在 CameraPreview 类中获取 SurfaceView,并设置它作为 Camera 的预览:
```java
public class CameraPreview extends SurfaceView implements SurfaceHolder.Callback {
private Camera mCamera;
public CameraPreview(Context context, Camera camera) {
super(context);
mCamera = camera;
// Install a SurfaceHolder.Callback so we get notified when the
// underlying surface is created and destroyed.
SurfaceHolder holder = getHolder();
holder.addCallback(this);
}
public void surfaceCreated(SurfaceHolder holder) {
// The Surface has been created, now tell the camera where to draw the preview.
try {
mCamera.setPreviewDisplay(holder);
mCamera.startPreview();
} catch (IOException e) {
Log.d(TAG, "Error setting camera preview: " + e.getMessage());
}
}
public void surfaceDestroyed(SurfaceHolder holder) {
// empty. Take care of releasing the Camera preview in your activity.
}
public void surfaceChanged(SurfaceHolder holder, int format, int w, int h) {
// If your preview can change or rotate, take care of those events here.
// Make sure to stop the preview before resizing or reformatting it.
if (holder.getSurface() == null){
// preview surface does not exist
return;
}
// stop preview before making changes
try {
mCamera.stopPreview();
} catch (Exception e){
// ignore: tried to stop a non-existent preview
}
// set preview size and make any resize, rotate or
// reformatting changes here
// start preview with new settings
try {
mCamera.setPreviewDisplay(holder);
mCamera.startPreview();
} catch (Exception e){
Log.d(TAG, "Error starting camera preview: " + e.getMessage());
}
}
}
```
3. 在 SurfaceView 上绘制时间水印,可以在 SurfaceView 的回调方法 surfaceChanged() 中获取 Canvas 对象,并在其中绘制水印:
```java
private void drawTimeWatermark(Canvas canvas) {
Paint paint = new Paint();
paint.setColor(Color.WHITE);
paint.setTextSize(50);
canvas.drawText(getCurrentTime(), 100, 100, paint);
}
private String getCurrentTime() {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return sdf.format(new Date());
}
public void surfaceChanged(SurfaceHolder holder, int format, int w, int h) {
if (holder.getSurface() == null){
return;
}
try {
mCamera.stopPreview();
} catch (Exception e){
// ignore: tried to stop a non-existent preview
}
try {
mCamera.setPreviewDisplay(holder);
mCamera.startPreview();
Canvas canvas = holder.lockCanvas();
drawTimeWatermark(canvas);
holder.unlockCanvasAndPost(canvas);
} catch (Exception e){
Log.d(TAG, "Error starting camera preview: " + e.getMessage());
}
}
```
这样就可以在 CameraPreview 中添加时间水印了。当然,还可以通过调整 Paint 对象的属性来改变水印的样式。
相关推荐
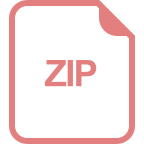














