我有一个来自十个类别各100个共1000个的信号数据,每个数据有512个特征点,存储为一个(300,1,512)的torch.tensor张量,现在我想将其输入一个深度DenseNet网络训练分类模型用于分类这些信号,请使用pytorch实现
时间: 2024-05-01 09:16:23 浏览: 95
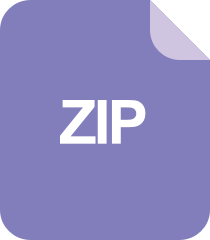
200个经典C程序源码(包括基础篇+数据结构篇+数值计算与趣味数学篇+图形篇+系统篇+常见试题解答篇).zip

以下是一个简单的实现:
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 定义深度DenseNet网络
class DenseNet(nn.Module):
def __init__(self):
super(DenseNet, self).__init__()
self.conv1 = nn.Conv1d(1, 64, kernel_size=3, padding=1)
self.dense1 = nn.Sequential(
nn.BatchNorm1d(64),
nn.ReLU(),
nn.Conv1d(64, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.dense2 = nn.Sequential(
nn.BatchNorm1d(96),
nn.ReLU(),
nn.Conv1d(96, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.dense3 = nn.Sequential(
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.dense4 = nn.Sequential(
nn.BatchNorm1d(160),
nn.ReLU(),
nn.Conv1d(160, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.dense5 = nn.Sequential(
nn.BatchNorm1d(192),
nn.ReLU(),
nn.Conv1d(192, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.dense6 = nn.Sequential(
nn.BatchNorm1d(224),
nn.ReLU(),
nn.Conv1d(224, 128, kernel_size=3, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.Conv1d(128, 32, kernel_size=1),
nn.BatchNorm1d(32),
nn.ReLU()
)
self.pool = nn.AdaptiveMaxPool1d(1)
self.fc = nn.Linear(32, 10)
def forward(self, x):
x = self.conv1(x)
x1 = self.dense1(x)
x1 = torch.cat([x, x1], 1)
x2 = self.dense2(x1)
x2 = torch.cat([x1, x2], 1)
x3 = self.dense3(x2)
x3 = torch.cat([x2, x3], 1)
x4 = self.dense4(x3)
x4 = torch.cat([x3, x4], 1)
x5 = self.dense5(x4)
x5 = torch.cat([x4, x5], 1)
x6 = self.dense6(x5)
x6 = torch.cat([x5, x6], 1)
x = self.pool(x6)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
# 定义数据集
class SignalDataset(torch.utils.data.Dataset):
def __init__(self, data):
self.data = data
def __getitem__(self, index):
return self.data[index], index // 100
def __len__(self):
return len(self.data)
# 加载数据
data = torch.load('data.pt')
dataset = SignalDataset(data)
dataloader = torch.utils.data.DataLoader(dataset, batch_size=32, shuffle=True)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(densenet.parameters(), lr=0.001)
# 训练模型
for epoch in range(10):
running_loss = 0.0
for i, (inputs, labels) in enumerate(dataloader, 0):
optimizer.zero_grad()
outputs = densenet(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 10 == 9:
print('[%d, %5d] loss: %.3f' % (epoch + 1, i + 1, running_loss / 10))
running_loss = 0.0
```
注意,这只是一个简单的实现,您可能需要根据您的数据进行一些调整。此外,您可能还需要使用更复杂的DenseNet结构,以获得更好的分类性能。
阅读全文
相关推荐
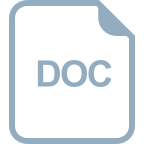
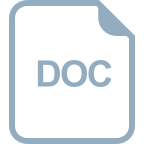



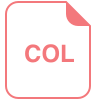
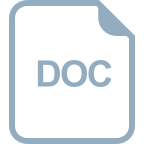
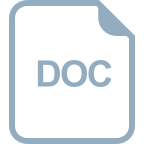
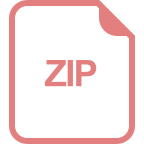
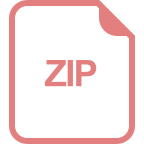
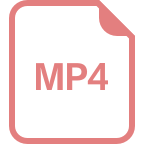
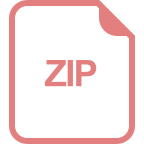
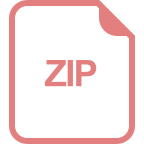
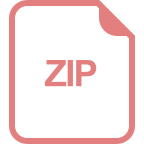
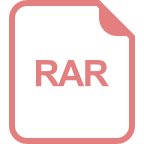
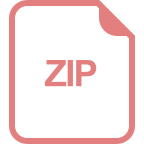