用MATLAB写一段代码,实现求取已知球心和半径的球的球面的点的坐标
时间: 2023-03-23 16:01:53 浏览: 91
好的,下面是用MATLAB实现求取已知球心和半径的球的球面点的坐标的代码:
```
% 假设球心为(x0,y0,z0),半径为r
x0 = 1;
y0 = 2;
z0 = 3;
r = 4;
% 生成球面点的坐标
theta = linspace(0, 2*pi, 101);
phi = linspace(0, pi, 51);
[theta, phi] = meshgrid(theta, phi);
x = r*sin(phi).*cos(theta) + x0;
y = r*sin(phi).*sin(theta) + y0;
z = r*cos(phi) + z0;
% 画出球面点的三维图像
figure
surf(x, y, z)
axis equal
```
这段代码使用了极坐标系下球面坐标的参数化公式,生成了球面点的坐标,并画出了球面点的三维图像。
相关问题
用matlab写一个函数 实现求取已知球心和半径的球的球面的100个采样点的坐标
好的,我可以回答这个问题。以下是用Matlab编写的函数,可以实现给定球心和半径的球面的100个采样点的坐标:
```
function [x,y,z] = getSpherePoints(center,radius)
% center: 3-element vector specifying the center of the sphere
% radius: scalar specifying the radius of the sphere
theta=linspace(0,2*pi,100); % divide the sphere into 100 vertical slices
phi=linspace(0,pi,100); % divide the sphere into 100 horizontal slices
[x,y,z]=meshgrid(radius*sin(phi).*cos(theta),radius*sin(phi).*sin(theta),radius*cos(phi));
% generate a grid of points on the surface of the sphere
x=x+center(1); % shift the coordinates so the center of the sphere is at the given center point
y=y+center(2);
z=z+center(3);
x=x(:); % convert to column vectors
y=y(:);
z=z(:);
end
```
使用这个函数,您可以提供球心和半径,并获得一个具有100个采样点的球面的x、y和z坐标。例如,如果您想要球心在原点,半径为1的球面的100个采样点,则可以运行以下代码:
```
[x,y,z]=getSpherePoints([0,0,0],1);
```
这将返回三个列向量,每个向量包含100个元素,分别是球面上对应点的x、y和z坐标。
在一个空间直角坐标系中,已知一个球的半径为300.4,和球面上三个点的坐标,求球心坐标,用matlab实现
可以使用Matlab的数学工具箱来计算球心坐标。假设球面上三个点的坐标分别为(x1, y1, z1),(x2, y2, z2),(x3, y3, z3)。
首先,利用球面上三个点的坐标计算球心的方向向量。可以使用以下公式:
```
v1 = [x1, y1, z1];
v2 = [x2, y2, z2];
v3 = [x3, y3, z3];
n = cross(v2 - v1, v3 - v1);
```
然后,对方向向量进行归一化处理:
```
n = n / norm(n);
```
最后,利用归一化后的方向向量和球面上任意一个点的坐标,以及球的半径,计算球心坐标:
```
r = 300.4;
v = v1 + r * n;
```
以下是完整的Matlab代码示例:
```matlab
% 三个球面上的点坐标
x1 = ...; % 第一个点的x坐标
y1 = ...; % 第一个点的y坐标
z1 = ...; % 第一个点的z坐标
x2 = ...; % 第二个点的x坐标
y2 = ...; % 第二个点的y坐标
z2 = ...; % 第二个点的z坐标
x3 = ...; % 第三个点的x坐标
y3 = ...; % 第三个点的y坐标
z3 = ...; % 第三个点的z坐标
% 计算球心的方向向量
v1 = [x1, y1, z1];
v2 = [x2, y2, z2];
v3 = [x3, y3, z3];
n = cross(v2 - v1, v3 - v1);
% 归一化方向向量
n = n / norm(n);
% 计算球心坐标
r = 300.4;
v = v1 + r * n;
% 输出球心坐标
disp(v);
```
请注意,以上代码中的省略号(...)需要替换为实际的坐标值。
相关推荐
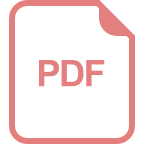












