java实验代码实现多态,线程,文件读写
时间: 2023-08-04 10:37:57 浏览: 100
好的,我来为您展示一下 Java 实验代码实现多态、线程和文件读写的示例代码。
多态实现示例代码:
```java
public class Shape {
public void draw() {
System.out.println("Drawing a shape");
}
}
public class Circle extends Shape {
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Square extends Shape {
public void draw() {
System.out.println("Drawing a square");
}
}
public class Main {
public static void main(String[] args) {
Shape shape1 = new Circle();
Shape shape2 = new Square();
shape1.draw();
shape2.draw();
}
}
```
线程实现示例代码:
```java
public class MyThread extends Thread {
public void run() {
for(int i = 1; i <= 5; i++) {
System.out.println("Thread " + Thread.currentThread().getId() + ": " + i);
}
}
}
public class Main {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyThread thread2 = new MyThread();
thread1.start();
thread2.start();
}
}
```
文件读写实现示例代码:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 写入文件
try {
FileWriter writer = new FileWriter("test.txt");
writer.write("Hello World!");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
// 读取文件
try {
File file = new File("test.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String data = scanner.nextLine();
System.out.println(data);
}
scanner.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
```
以上就是 Java 实验代码实现多态、线程和文件读写的示例代码,希望能对您有所帮助!
阅读全文
相关推荐
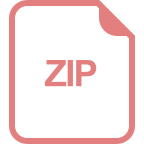
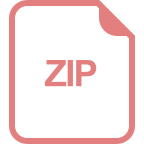
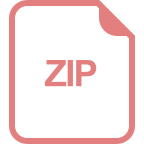
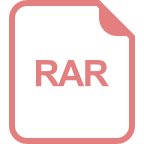
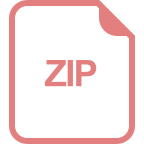
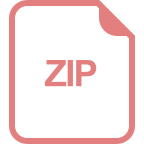
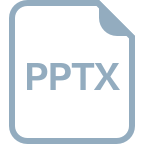
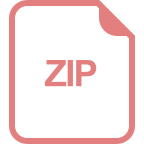
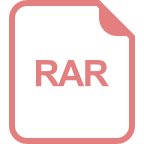
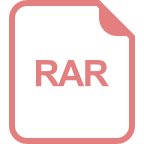
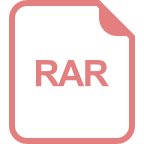
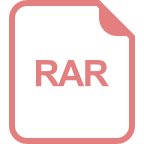
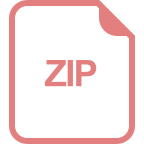
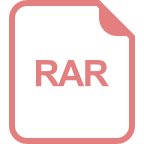
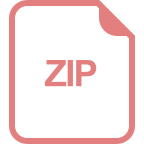
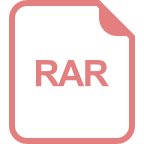
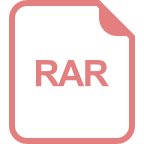