Katalon写一个Groovy脚本,java8利用java-diff-util实现两个文件夹对比,并将比较结果以文件为单位出力成各自的HTML文件,HTML中要体现差异数据在两个文件中所对应的行数以及内容分别是什么。要求:当文件在两个文件夹中都存在时,能识别隔行数据,将两个文件有差异的数据都出力到HTML中,将两个文件的差异数据以逗号隔开,并将有差异的字段高亮显示出来;当文件只在一个文件夹中存在的文件,将这个信息出力到HTML中
时间: 2024-03-03 16:54:03 浏览: 18
好的,以下是一个可能的实现方案。需要注意的是,需要在项目中引入 java-diff-utils 和 jsoup 两个库。
```groovy
import difflib.*
import org.jsoup.Jsoup
import org.jsoup.nodes.Document
import org.jsoup.nodes.Element
// 定义两个文件夹的路径
def folder1Path = "/path/to/folder1"
def folder2Path = "/path/to/folder2"
// 获取两个文件夹中的所有文件
def folder1 = new File(folder1Path)
def folder2 = new File(folder2Path)
def allFiles = (folder1.listFiles() as List) + (folder2.listFiles() as List)
def uniqueFiles = allFiles.unique()
// 定义输出文件夹的路径
def outputFolderPath = "/path/to/output/folder"
// 遍历所有文件,进行对比
uniqueFiles.each { file ->
if (file.isFile()) {
// 获取文件名和文件路径
def fileName = file.getName()
def filePath1 = folder1Path + "/" + fileName
def filePath2 = folder2Path + "/" + fileName
def outputPath = outputFolderPath + "/" + fileName + ".html"
// 判断文件是否存在于两个文件夹中
def file1 = new File(filePath1)
def file2 = new File(filePath2)
if (file1.exists() && file2.exists()) {
// 对比两个文件的内容
def lines1 = file1.readLines()
def lines2 = file2.readLines()
def patch = DiffUtils.diff(lines1, lines2)
// 生成 HTML 文件
def doc = Jsoup.parse("<html></html>")
def body = doc.appendElement("body")
body.appendElement("h1").text(fileName)
body.appendElement("p").text("文件存在于两个文件夹中")
def table = body.appendElement("table")
table.appendElement("tr").appendElements(["th", "th", "th"]).forEach { th ->
th.attr("style", "border: 1px solid black; padding: 5px;")
}
table.appendElement("tr").appendElements(["td", "td", "td"]).forEach { td ->
td.attr("style", "border: 1px solid black; padding: 5px;")
}
patch.getDeltas().each { delta ->
delta.getOriginal().each { line ->
def tr = table.appendElement("tr")
tr.appendElement("td").text("- " + line)
tr.appendElement("td").text("")
tr.appendElement("td").text("")
}
delta.getRevised().each { line ->
def tr = table.appendElement("tr")
tr.appendElement("td").text("")
tr.appendElement("td").text("+ " + line)
tr.appendElement("td").text("")
}
delta.getOriginal().zip(delta.getRevised()).each { pair ->
def originalLine = pair[0]
def revisedLine = pair[1]
def tr = table.appendElement("tr")
if (originalLine != revisedLine) {
tr.appendElement("td").text("- " + originalLine).attr("style", "color: red;")
tr.appendElement("td").text("+ " + revisedLine).attr("style", "color: green;")
tr.appendElement("td").text("")
} else {
tr.appendElement("td").text(originalLine)
tr.appendElement("td").text(revisedLine)
tr.appendElement("td").text("")
}
}
}
def html = doc.outerHtml()
// 写入文件
new File(outputPath).write(html)
} else {
// 文件只存在于一个文件夹中
def doc = Jsoup.parse("<html></html>")
def body = doc.appendElement("body")
body.appendElement("h1").text(fileName)
body.appendElement("p").text("文件只存在于一个文件夹中")
body.appendElement("p").text("文件路径:" + (file1.exists() ? filePath1 : filePath2))
def html = doc.outerHtml()
// 写入文件
new File(outputPath).write(html)
}
}
}
```
这个脚本会遍历两个文件夹中的所有文件,并逐个进行对比。如果一个文件只存在于一个文件夹中,那么会生成一份 HTML 文件,里面记录下这个信息;如果一个文件存在于两个文件夹中,那么会生成一份 HTML 文件,里面记录下这个文件两个版本的差异。
在 HTML 文件中,差异数据会以表格的形式出现,每个差异数据都对应着两个文件中的一行。如果两个文件的某一行是一样的,那么这一行就不会被标记为差异数据。如果两个文件的某一行不一样,那么这一行会被标记为差异数据,并且被高亮显示出来。如果两个文件的某一行在另一个文件中没有对应的行,那么这一行也会被单独的标记为差异数据,但是不会高亮显示。
相关推荐
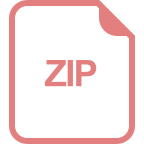
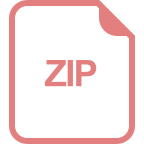


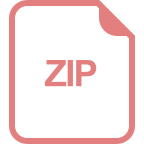
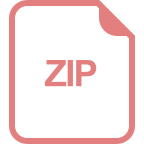
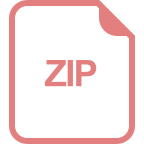
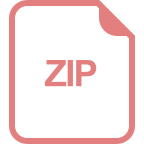
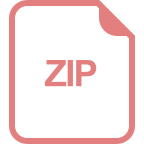
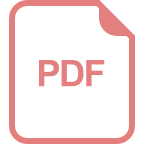
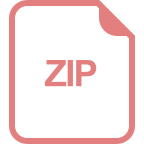
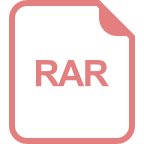
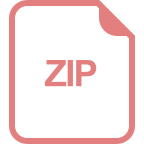
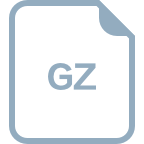
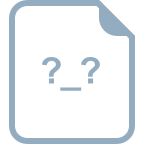
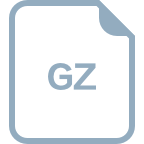
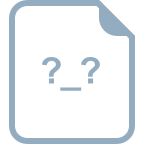