jtable单选
时间: 2023-08-14 14:06:32 浏览: 172
在 JTable 中实现单选功能需要使用 ListSelectionModel 和 SelectionMode。具体步骤如下:
1. 获取表格的选择模型:
```
ListSelectionModel selectionModel = table.getSelectionModel();
```
2. 设置选择模式为单选:
```
selectionModel.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
```
3. 监听选择事件,获取选中的行号:
```
selectionModel.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent event) {
if (!event.getValueIsAdjusting()) {
int selectedRow = table.getSelectedRow();
//TODO: 处理选中行的操作
}
}
});
```
通过以上步骤,即可在 JTable 中实现单选功能。
相关问题
怎么设置jtable单选框只能单选
要设置JTable单选框只能单选,需要使用TableCellRenderer和TableCellEditor接口的实现类进行自定义。首先需要创建一个单选的渲染器,可以使用JRadioButton来实现。然后创建一个单选的编辑器,当用户点击单元格时,它将在编辑模式下显示。在实现编辑器时,应将值更改为用户选择的单选按钮的值。
具体实现可以参考以下代码:
```
public class RadioButtonRenderer extends JRadioButton implements TableCellRenderer {
public RadioButtonRenderer() {
setOpaque(true);
}
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
if (value != null) {
setSelected((Boolean) value);
}
return this;
}
}
public class RadioButtonEditor extends DefaultCellEditor implements ItemListener {
private JRadioButton button;
public RadioButtonEditor(JCheckBox checkBox) {
super(checkBox);
}
public Component getTableCellEditorComponent(JTable table, Object value, boolean isSelected, int row, int column) {
if (value == null) {
return null;
}
button = (JRadioButton) value;
button.addItemListener(this);
return (Component) value;
}
public Object getCellEditorValue() {
button.removeItemListener(this);
return button;
}
public void itemStateChanged(ItemEvent e) {
super.fireEditingStopped();
}
}
```
然后在使用JTable时,可以直接为需要单选框的列设置渲染器和编辑器即可:
```
DefaultTableModel tm = new DefaultTableModel(new Object[][]{}, new String[]{"Name", "Gender", "Age"});
JTable table = new JTable(tm);
table.getColumnModel().getColumn(1).setCellRenderer(new RadioButtonRenderer());
table.getColumnModel().getColumn(1).setCellEditor(new RadioButtonEditor(new JCheckBox()));
```
这样就可以将JTable的某一列变成单选框,并且只能选择一个选项了。
怎么设置jtable单选框只能单选附上代码和注释
好的,我会尽力回答你的问题。以下是示例代码和注释,可以参考一下:
```java
// 创建具有单选框的 JTable,使用 DefaultTableModel 作为数据模型
JTable table = new JTable(new DefaultTableModel(data, columnNames) {
// 重写 isCellEditable() 方法,使单元格不可编辑
public boolean isCellEditable(int row, int column) {
return false;
}
});
// 获取表格的选择模型,并设置为单选模式
ListSelectionModel selectionModel = table.getSelectionModel();
selectionModel.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
// 添加 ListSelectionListener,当用户选择某个单元格时触发
selectionModel.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent event) {
// 处理用户选择单元格的事件
}
});
```
以上代码中,我们先创建一个带有单选框的 JTable,并使用 DefaultTableModel 作为数据模型。然后,我们设置表格选择模型为单选模式,并添加 ListSelectionListener 来处理用户选择单元格的事件。此外,我们还重写了 isCellEditable() 方法,使单元格不可编辑。
阅读全文
相关推荐








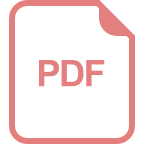
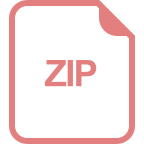
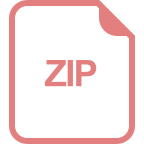
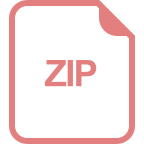
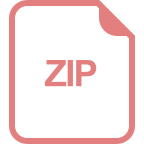
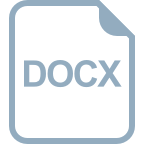