toggleCollapsed(toggle?: boolean) { this.isCollapsed = toggle ?? !this.isCollapsed },
时间: 2024-05-20 21:10:27 浏览: 157
这是一个 TypeScript 中的方法,用于切换一个布尔类型的变量 `isCollapsed`,来控制一个组件的收缩或展开状态。它的形参 `toggle` 是一个可选的布尔类型,表示切换的状态,如果传入了 `toggle` 的值,则直接使用该值作为 `isCollapsed` 的值;否则,使用取反操作符 `!` 将 `isCollapsed` 取反作为新的值。其中,`??` 表示空值合并操作符,如果 `toggle` 的值是 `null` 或 `undefined`,则使用后面的默认值。
相关问题
这串代码的作用import { VuexModule, Module, Mutation, Action, getModule } from 'vuex-module-decorators' import { getSidebarStatus, setSidebarStatus } from '@/utils/cookies' import store from '@/store' import enumsList from '@/utils/enums' export enum DeviceType { Mobile, Desktop, } export interface IAppState { device: DeviceType sidebar: { opened: boolean withoutAnimation: boolean }, enumsList: object } @Module({ dynamic: true, store, name: 'app' }) class App extends VuexModule implements IAppState { public sidebar = { opened: getSidebarStatus() !== 'closed', withoutAnimation: false } public device = DeviceType.Desktop public enumsList = enumsList @Mutation private TOGGLE_SIDEBAR(withoutAnimation: boolean) { this.sidebar.opened = !this.sidebar.opened this.sidebar.withoutAnimation = withoutAnimation if (this.sidebar.opened) { setSidebarStatus('opened') } else { setSidebarStatus('closed') } } @Mutation private CLOSE_SIDEBAR(withoutAnimation: boolean) { this.sidebar.opened = false this.sidebar.withoutAnimation = withoutAnimation setSidebarStatus('closed') } @Mutation private TOGGLE_DEVICE(device: DeviceType) { this.device = device } @Action public ToggleSideBar(withoutAnimation: boolean) { this.TOGGLE_SIDEBAR(withoutAnimation) } @Action public CloseSideBar(withoutAnimation: boolean) { this.CLOSE_SIDEBAR(withoutAnimation) } @Action public ToggleDevice(device: DeviceType) { this.TOGGLE_DEVICE(device) } } export const AppModule = getModule(App)
这段代码定义了一个 Vuex 模块,其中包含了一些状态和修改状态的方法。具体来说,它定义了一个名为 App 的模块,其中包含了一个 IAppState 接口和一个 App 类。IAppState 定义了应用程序的状态,包括设备类型、侧边栏状态和枚举列表;而 App 类则实现了这个接口,并定义了一些 mutation 和 action,用于修改和处理这些状态。
在这个模块中,TOGGLE_SIDEBAR、CLOSE_SIDEBAR 和 TOGGLE_DEVICE 这些 mutation 分别用于切换侧边栏状态和设备类型,而 ToggleSideBar、CloseSideBar 和 ToggleDevice 这些 action 则是对应的方法,用于触发 mutation。同时,还定义了一个 enumsList 对象,用于存储应用程序中的枚举列表。最后,通过 getModule 函数将这个模块导出,并注册到 Vuex 的 store 中。
vue.runtime.esm.js?c320:4605 [Vue warn]: Invalid prop: type check failed for prop "collapse". Expected Boolean, got Function
This error message means that the "collapse" prop is expecting a boolean value, but it is receiving a function instead.
To fix this error, you should check where the "collapse" prop is being used and ensure that it is being passed a boolean value. If the value needs to be dynamic or computed, make sure that the function returns a boolean value.
For example, if you have a component that uses the "collapse" prop:
<template>
<div>
<button @click="toggleCollapse">{{ collapse ? 'Expand' : 'Collapse' }}</button>
<div v-if="!collapse">
<p>Some content here</p>
</div>
</div>
</template>
<script>
export default {
props: {
collapse: {
type: Boolean,
default: false
}
},
methods: {
toggleCollapse() {
this.$emit('update:collapse', !this.collapse);
}
}
}
</script>
In this example, the "collapse" prop is used to conditionally render the content inside the component. The "toggleCollapse" method is used to toggle the value of the "collapse" prop.
If you are passing a function instead of a boolean value to the "collapse" prop, you will need to update the code to pass a boolean value instead:
<template>
<my-component :collapse="isCollapsed"></my-component>
</template>
<script>
export default {
data() {
return {
isCollapsed: false
}
},
methods: {
toggleCollapse() {
this.isCollapsed = !this.isCollapsed;
}
}
}
</script>
In this example, we are passing the "isCollapsed" data property to the "collapse" prop. The "toggleCollapse" method is used to update the value of "isCollapsed" which will then update the "collapse" prop.
阅读全文
相关推荐
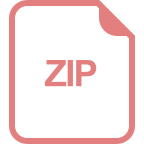
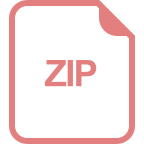
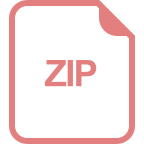













