java字符串转json对象
时间: 2023-07-19 13:15:24 浏览: 85
在 Java 中,我们可以使用第三方库,比如 Jackson 或者 Gson 来将字符串转换为 JSON 对象。以下是使用 Jackson 和 Gson 将字符串转换为 JSON 对象的示例代码:
使用 Jackson:
```java
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = "{\"name\": \"John\", \"age\": 30, \"city\": \"New York\"}";
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode.get("name")); // output: "John"
System.out.println(jsonNode.get("age")); // output: 30
System.out.println(jsonNode.get("city")); // output: "New York"
} catch (JsonProcessingException e) {
e.printStackTrace();
}
```
使用 Gson:
```java
Gson gson = new Gson();
String jsonString = "{\"name\": \"John\", \"age\": 30, \"city\": \"New York\"}";
JsonElement jsonElement = gson.fromJson(jsonString, JsonElement.class);
JsonObject jsonObject = jsonElement.getAsJsonObject();
System.out.println(jsonObject.get("name")); // output: "John"
System.out.println(jsonObject.get("age")); // output: 30
System.out.println(jsonObject.get("city")); // output: "New York"
```
注意:以上代码中的字符串必须是有效的 JSON 格式,否则会抛出异常。
相关推荐
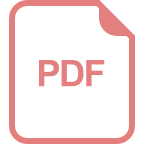
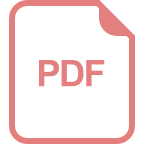
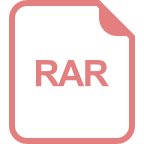
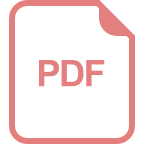
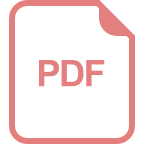
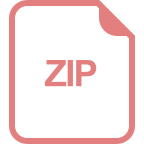
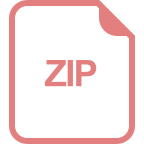
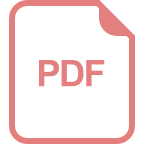
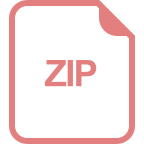
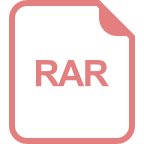
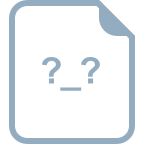
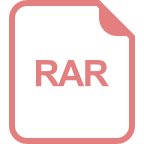
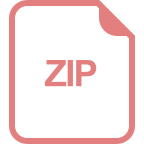
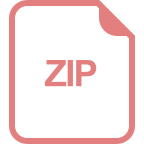
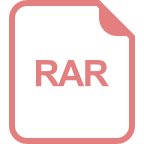