在ros系统中使用python编程使用摄像头实时识别apriltag二维码并保存识别的数据,并保存为txt文件
时间: 2024-03-17 11:39:48 浏览: 78
好的,这是一个比较复杂的问题,需要分几步来回答。首先,我们需要安装apriltag的ROS包,可以使用以下命令在终端中进行安装:
```
sudo apt-get install ros-<distro>-apriltag-ros
```
其中,`<distro>`替换为你使用的ROS发行版,比如`melodic`或`noetic`。
接下来,我们需要使用Python编写代码来实现摄像头实时识别apriltag二维码。可以使用`cv2`库来读取摄像头数据,并使用`apriltag_ros`包中的`Detector`类来进行apriltag识别。具体代码如下:
```python
import cv2
from apriltag_ros.msg import AprilTagDetectionArray
def callback(data):
for detection in data.detections:
print('AprilTag ID:', detection.id[0])
print('AprilTag pose:', detection.pose.pose)
rospy.init_node('apriltag_detector')
rospy.Subscriber('/tag_detections', AprilTagDetectionArray, callback)
cap = cv2.VideoCapture(0)
while not rospy.is_shutdown():
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
detections = detector.detect(gray)
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
其中,回调函数`callback`会在每次识别到apriltag时被调用,将apriltag的ID和位姿信息打印出来。`rospy.Subscriber`用来订阅apriltag识别结果的ROS话题,`/tag_detections`是apriltag_ros包中默认的话题。`cv2.VideoCapture`用来打开摄像头,`cv2.imshow`用来显示摄像头的画面。按下`q`键可以退出程序。
最后,我们需要将识别到的apriltag数据保存为txt文件。可以在回调函数中添加以下代码:
```python
with open('apriltag_data.txt', 'a') as f:
f.write('{}\n'.format(detection.pose.pose))
```
这样就可以将每次识别到的apriltag位姿信息保存到名为`apriltag_data.txt`的文件中。
希望这些代码可以帮助到你。如果你有进一步的问题,请随时问我。
阅读全文
相关推荐














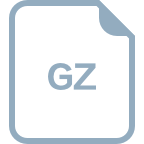
