rsa非对称密码算法实验java实现
时间: 2023-08-16 12:07:33 浏览: 122
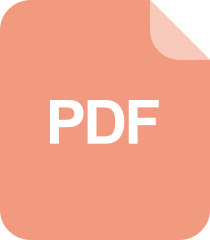
java 非对称加密算法RSA实现详解

好的,我可以为您提供一个简单的RSA非对称密码算法的Java实现,代码如下:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class RSA {
private BigInteger privateKey;
private BigInteger publicKey;
private BigInteger modulus;
private int bitLength = 1024;
private SecureRandom random;
// 构造函数
public RSA() {
random = new SecureRandom();
BigInteger p = BigInteger.probablePrime(bitLength, random);
BigInteger q = BigInteger.probablePrime(bitLength, random);
BigInteger phi = (p.subtract(BigInteger.ONE)).multiply(q.subtract(BigInteger.ONE));
modulus = p.multiply(q);
publicKey = BigInteger.probablePrime(bitLength / 2, random);
while (phi.gcd(publicKey).compareTo(BigInteger.ONE) > 0 && publicKey.compareTo(phi) < 0) {
publicKey.add(BigInteger.ONE);
}
privateKey = publicKey.modInverse(phi);
}
// 获取私钥
public BigInteger getPrivateKey() {
return privateKey;
}
// 获取公钥
public BigInteger getPublicKey() {
return publicKey;
}
// 获取模数
public BigInteger getModulus() {
return modulus;
}
// 加密
public byte[] encrypt(byte[] message) {
return (new BigInteger(message)).modPow(publicKey, modulus).toByteArray();
}
// 解密
public byte[] decrypt(byte[] message) {
return (new BigInteger(message)).modPow(privateKey, modulus).toByteArray();
}
}
```
使用该RSA类进行加密和解密的示例代码如下:
```java
public static void main(String[] args) {
RSA rsa = new RSA();
// 明文
String message = "Hello, world!";
// 加密
byte[] encryptedMessage = rsa.encrypt(message.getBytes());
// 解密
byte[] decryptedMessage = rsa.decrypt(encryptedMessage);
System.out.println("明文: " + message);
System.out.println("加密后的密文: " + new String(encryptedMessage));
System.out.println("解密后的明文: " + new String(decryptedMessage));
}
```
注意:该实现只是一个简单的示例,实际使用中需要加入更多的安全措施,比如添加数字签名等。
阅读全文
相关推荐
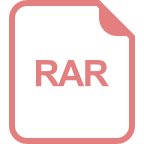
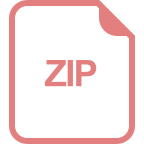















