python图片批量转word
时间: 2023-07-27 15:05:56 浏览: 158
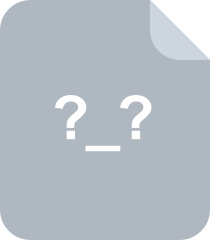
使用Python对word批量插入图片
你可以使用 Python 中的 PIL(Python Imaging Library)和 python-docx 库来实现将图片批量转换为 Word 文档。下面是一个简单的代码示例:
```python
from PIL import Image
from docx import Document
from docx.shared import Inches
# 创建一个空白文档
document = Document()
# 遍历所有图片
for i in range(1, 11):
# 打开图片
image = Image.open(f"image_{i}.jpg")
# 将图片插入到文档中
document.add_picture(f"image_{i}.jpg", width=Inches(6), height=Inches(4.5))
# 保存文档
document.save("images.docx")
```
这段代码将会遍历当前目录下的 `image_1.jpg` 到 `image_10.jpg` 这 10 张图片,并将它们插入到一个名为 `images.docx` 的 Word 文档中。你可以根据需要修改代码以适应你的具体需求。
阅读全文
相关推荐
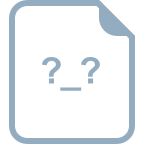



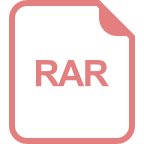
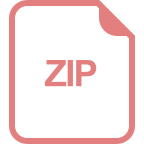
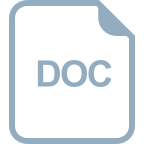
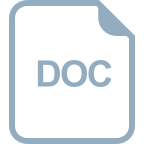
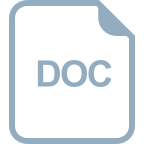
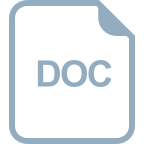
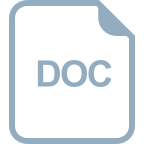




