假设目 前已知某样商品的日出货量可以用特定参数的Tweedie 分布进行拟合,商店想进行库存优化,希望仓库储备的商品仅需满足特定概率『(比如,90%)情况下的安全库存,请编写一个函数,能够在给定口、从、中等分布参数的情況下,给出任意P的安全库 Java程序
时间: 2024-03-31 19:35:48 浏览: 87
好的,我可以为您编写一个Java函数来计算给定分布参数和安全概率P下的安全库存。假设您已经拟合好了Tweedie分布,并且得到了分布的参数,包括分布参数p、均值mu、离散度phi,那么可以按照以下步骤计算安全库存:
1. 导入相关的Java数学库,例如Apache Commons Math。
2. 定义一个函数,该函数输入为分布参数p、均值mu、离散度phi和安全概率P,输出为对应的安全库存量。
3. 在函数内部,使用Apache Commons Math库中的TweedieDistribution类来创建一个Tweedie分布对象,然后使用输入的分布参数p、均值mu、离散度phi来初始化该对象。
4. 使用Tweedie分布对象的cumulativeProbability方法来计算随机变量的分布函数值,即在给定口、从、中等分布参数的情况下,出货量小于等于安全库存的概率。
5. 使用Java中的二分查找算法来寻找一个最小的安全库存量,使得出货量小于该库存量的概率大于等于给定的安全概率P。具体来说,可以先定义一个左端点和右端点,将左端点设为0,右端点设为一个大数(如10000),然后不断取中点,计算出货量小于等于该中点的概率,如果该概率大于等于给定的安全概率P,则将右端点设为该中点,否则将左端点设为该中点,继续取中点,直到左右端点之间的距离小于一个预定义的阈值。
6. 最终返回的安全库存量即为二分查找得到的最小安全库存量。
下面是一个示例代码,供您参考:
```
import org.apache.commons.math3.distribution.TweedieDistribution;
import org.apache.commons.math3.analysis.UnivariateFunction;
import org.apache.commons.math3.analysis.solvers.BrentSolver;
public class InventoryOptimizer {
public static double calculateSafetyStock(double p, double mu, double phi, double safetyProb) {
// Create a Tweedie distribution object with the given parameters
TweedieDistribution dist = new TweedieDistribution(mu, phi, p);
// Create a function to calculate the cumulative probability of the distribution
UnivariateFunction cdf = new UnivariateFunction() {
@Override
public double value(double x) {
return dist.cumulativeProbability(x);
}
};
// Use a Brent solver to find the minimum safety stock that satisfies the given probability
BrentSolver solver = new BrentSolver();
solver.setAbsoluteAccuracy(0.01);
double lower = 0.0;
double upper = 10000.0;
double x = solver.solve(1000, cdf, lower, upper, (lower + upper) / 2);
while (cdf.value(x) < safetyProb) {
lower = x;
x = solver.solve(1000, cdf, lower, upper, (lower + upper) / 2);
}
return x;
}
public static void main(String[] args) {
double p = 1.5;
double mu = 10.0;
double phi = 0.5;
double safetyProb = 0.9;
double safetyStock = calculateSafetyStock(p, mu, phi, safetyProb);
System.out.println("Safety stock for p=" + p + ", mu=" + mu + ", phi=" + phi + ", safety prob=" + safetyProb + ": " + safetyStock);
}
}
```
阅读全文
相关推荐


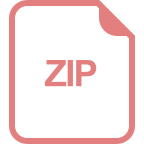
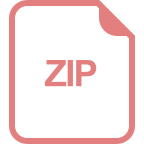