用python 将excel的表格转化为固定格式的文件
时间: 2024-04-08 19:29:29 浏览: 253
你可以使用 Python 中的 pandas 库将 Excel 表格转换为固定格式的文件。以下是一个简单的示例代码,演示如何将 Excel 表格转换为 CSV 文件:
```python
import pandas as pd
# 读取 Excel 表格
df = pd.read_excel('input.xlsx')
# 将数据保存为 CSV 文件
df.to_csv('output.csv', index=False)
```
在这个示例中,我们使用 pandas 的 `read_excel` 函数读取名为 `input.xlsx` 的 Excel 文件,并将数据存储在 pandas 的 DataFrame 对象 `df` 中。然后,我们使用 DataFrame 的 `to_csv` 方法将数据保存为名为 `output.csv` 的 CSV 文件。`index=False` 参数用于禁止保存索引列。
你可以根据需要调整文件的输入和输出路径,以及选择其他的固定格式(如 JSON、XML 等)。pandas 提供了多种文件格式的读写功能,你可以根据具体需求进行选择和调整。
相关问题
使用python把EXCEL表格转化成图
您好,可以使用 Python 中的 pandas 和 matplotlib 库来将 Excel 表格转换为图表。下面是一个简单的示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取 Excel 表格
df = pd.read_excel('your_excel_file.xlsx')
# 绘制折线图
plt.plot(df['x'], df['y'])
# 显示图表
plt.show()
```
在代码中,`read_excel()` 方法用于读取 Excel 表格,`plot()` 方法用于绘制折线图,`show()` 方法用于显示图表。您需要将代码中的 `your_excel_file.xlsx` 替换为您自己的 Excel 文件路径,以及 `x` 和 `y` 替换为您的 Excel 表格中要绘制的数据列。此外,您还可以使用其他 matplotlib 方法来自定义图表的样式和属性。
python编程将excel表格转化为网页表格
在Python中,我们可以利用一些库如pandas、openpyxl和html、jinja2等来实现Excel表格数据转换为HTML网页表格的功能。以下是一个简单的步骤概述:
1. 首先,安装必要的库:如果你还没有安装,可以使用pip安装`pandas`, `openpyxl`, 和 `jinja2`,以及用于渲染模板的`MarkupSafe`库。
```bash
pip install pandas openpyxl jinja2 MarkupSafe
```
2. 使用pandas读取Excel文件:
```python
import pandas as pd
# 加载Excel文件
df = pd.read_excel('your_file.xlsx')
```
3. 创建HTML模板:
```python
from jinja2 import Template
# 创建一个Jinja2模板
template = Template('''\
<table>
<tr>
{% for col in header %}
<th>{{ col }}</th>
{% endfor %}
</tr>
{% for row in data %}
<tr>
{% for cell in row %}
<td>{{ cell }}</td>
{% endfor %}
</tr>
{% endfor %}
</table>
''')
```
4. 将DataFrame内容渲染到模板中:
```python
# 获取表头(header)和数据(data)
header = df.columns.tolist()
data = df.values.tolist()
# 渲染模板并创建HTML字符串
html_table = template.render(header=header, data=data)
```
5. 最后,你可以选择保存到文件或直接返回HTML字符串,如果需要显示在浏览器中:
```python
with open('output.html', 'w') as f:
f.write(html_table)
# 或者直接返回给用户
print(html_table)
```
阅读全文
相关推荐
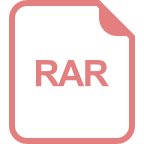













