使用注解的方式编写sql语句实现springboot文件上传下载
时间: 2024-01-27 18:03:47 浏览: 69
首先,我们需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
然后,我们需要创建一个实体类来映射上传文件的信息,例如:
```java
@Entity
@Table(name = "file")
public class File {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String fileName;
private String fileType;
private Long fileSize;
private String fileUrl;
//省略getter和setter方法
}
```
接下来,我们需要创建一个接口来定义上传和下载文件的方法,例如:
```java
public interface FileService {
//上传文件
File upload(MultipartFile file);
//根据文件id下载文件
Resource download(Long fileId);
}
```
然后,我们需要实现该接口,例如:
```java
@Service
public class FileServiceImpl implements FileService {
@Autowired
private FileRepository fileRepository;
@Value("${file.upload-dir}")
private String uploadDir;
@Override
public File upload(MultipartFile file) {
try {
File newFile = new File();
newFile.setFileName(file.getOriginalFilename());
newFile.setFileType(file.getContentType());
newFile.setFileSize(file.getSize());
//设置文件URL
String fileName = UUID.randomUUID().toString();
String fileUrl = uploadDir + File.separator + fileName;
newFile.setFileUrl(fileUrl);
File save = fileRepository.save(newFile);
//保存文件
Path path = Paths.get(fileUrl);
Files.copy(file.getInputStream(), path, StandardCopyOption.REPLACE_EXISTING);
return save;
} catch (IOException e) {
throw new RuntimeException("文件上传失败!", e);
}
}
@Override
public Resource download(Long fileId) {
Optional<File> optionalFile = fileRepository.findById(fileId);
if (optionalFile.isPresent()) {
File file = optionalFile.get();
Path path = Paths.get(file.getFileUrl());
Resource resource;
try {
resource = new UrlResource(path.toUri());
} catch (MalformedURLException e) {
throw new RuntimeException("文件下载失败!", e);
}
return resource;
} else {
throw new RuntimeException("文件不存在!");
}
}
}
```
接着,我们需要创建一个控制器来处理上传和下载文件的请求,例如:
```java
@RestController
@RequestMapping("/file")
public class FileController {
@Autowired
private FileService fileService;
//上传文件
@PostMapping("/upload")
public ResponseEntity<File> upload(@RequestParam("file") MultipartFile file) {
File save = fileService.upload(file);
return ResponseEntity.ok(save);
}
//下载文件
@GetMapping("/download/{fileId}")
public ResponseEntity<Resource> download(@PathVariable Long fileId) {
Resource resource = fileService.download(fileId);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
}
```
最后,我们需要在application.properties文件中配置上传文件的路径,例如:
```properties
file.upload-dir=/tmp/upload
```
这样,我们就通过注解的方式编写了一个实现文件上传和下载的 Spring Boot 应用。
阅读全文
相关推荐

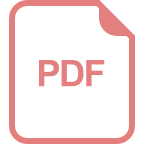
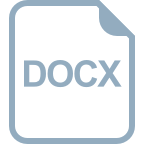





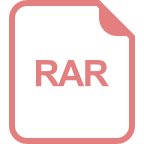
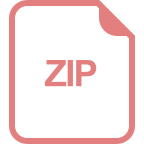
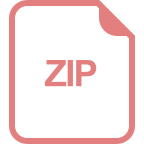
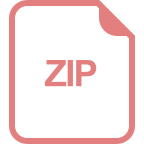
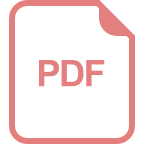
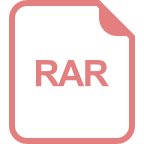
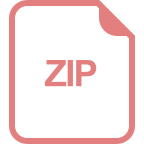
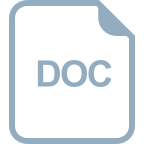